WebSockets is a protocol that enables the browser and server to interact with each other, it is distinct from the HTTP protocol, as it enables full-duplex communication. However, the WebSocket protocol is not supported for all browsers, especially not legacy versions. This problem is addressed by SockJS, as it supports a WebSocket-like protocol even when WebSockets is not supported. Long-polling used to be a workaround way of enabling full-duplex communication, but now that WebSocket exists, it is the most common tool for full-duplex communication.
SockJS is a JavaScript library that enables full-duplex communication. It works cross-browser, allows for low latency communication, between client and server. If WebSockets is not enabled by the browser being used, it will fall back to other means of communication, but with SocksJS it is still a WebSocket-like abstraction.
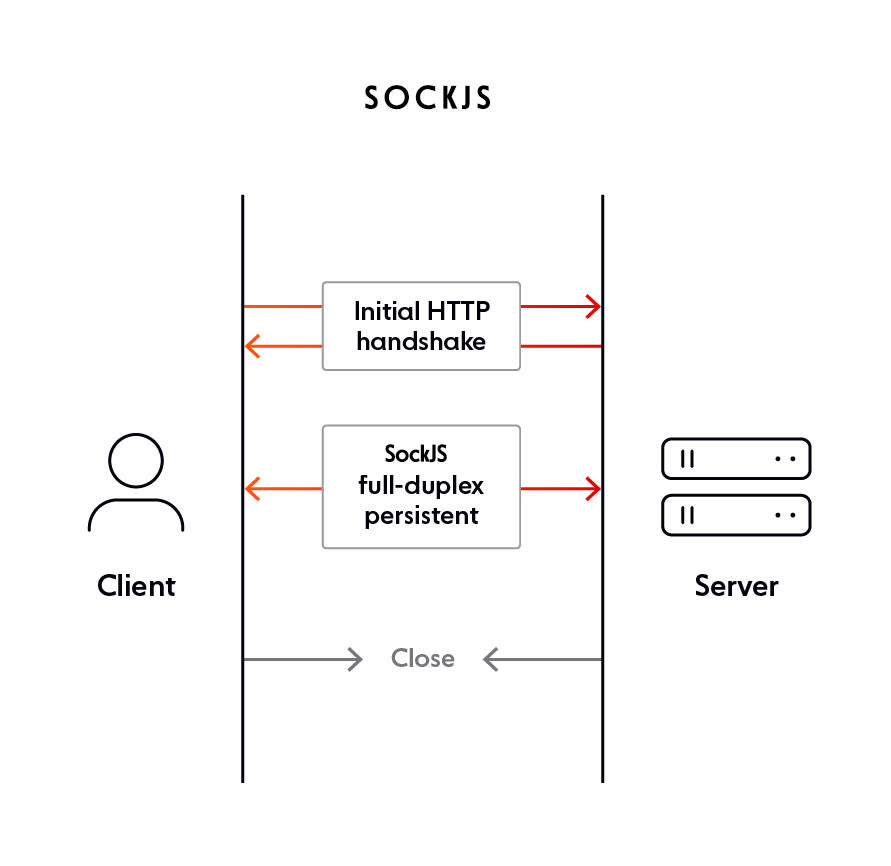
Figure 1: SockJS client-server connection diagram
The philosophy behind SockJS is the following: The API should follow the HTML5 WebSockets API. A stream protocol should be supported for each major browser. Support for cross-domain streaming transports. Support for cookies, which enables cookie-based sticky sessions. Where WebSockets is not enabled, in older browsers or when users are behind corporate firewalls, polling should be used.
SockJS has support for various programming languages, including JavaScript, NodeJS, Erlang, Python, Java, Scala, Rust, all found on the Github repository.
Getting started
In order to establish a socket-connection, the client sends a handshake request to a server, which in turn returns a handshake response. After the handshake is finished, a switch happens to the bidirectional binary protocol. Once the connection is established, the two parties can send text frames to each other. Each transmission is called a message, where it is possible for longer messages to be split into multiple messages, which ends with the FIN bit.
var sock = new SockJS('https://mydomain.com/my_prefix');
sock.onopen = function() {
console.log('open');
sock.send('test');
};
sock.onmessage = function(e) {
console.log('message', e.data);
sock.close();
};
sock.onclose = function() {
console.log('close');
};
To start a socket-connection in the client, you can create a new SockJS class by sending the URL to the constructor. Once the handshake between client and server has finished, and the protocol has switched over to WebSockets, the onopen function is triggered. The purpose of the onopen function is to let both parties know that the connection has been established, and both parties can prepare to receive messages. The onmessage function is run whenever a message is being received, to begin message processing. In order to know that the connection has been closed, the onclose function is called. The send function is run whenever the client sends a message to the receiving server.
SockJS Class
var sockjs = new SockJS(url, _reserved, options);
SockJS can be initiated similarly to regular WebSockets. The constructor takes one or more inputs, including the URL. The options argument can contain the following properties:
SessionId — provided either by a function or number to distinguish between connections.
Timeout number — sets the minimum timeout in seconds and determines when the connection should be closed.
Transports — a string or array of strings; useful to disable certain transportation options.
Benefits of using SockJS
A benefit of using SockJS compared to WebSockets is that SockJS will fall back to other means of full-duplex communication if WebSockets is not supported for the browser being used. This is particularly useful if you have to support older browsers, such as Internet Explorer, as IE 6, 7, 8, and 9 don’t support WebSockets — while accounting for 5% of browser usage in 2020.
Another benefit of using SockJS is that its syntax is identical to the WebSockets API, allowing you to switch to and from a WebSocket setup.
Downsides of using SockJS
Using SockJS may not be necessary if your application does not have to support legacy browser versions. Internet Explorer is not here to stay, as Microsoft has announced that it will stop supporting IE10 for their online services like Office 365, OneDrive, Outlook, and more on August 17th, 2021, thus making SockJS and similar libraries obsolete.
A study comparing the performance of different WebSocket-like frameworks, including plain WebSocket, Socket.IO, and SockJS, found that WebSocket can be 1.7 times faster than SockJS (and 3.7 times faster than Socket.IO). This makes sense, as support for multiple protocols adds overhead when WebSockets is not available. The study also found that SockJS does not scale well and doesn’t handle the same level of concurrency as Socket.IO or plain WebSocket.
Read more
Recommended Articles
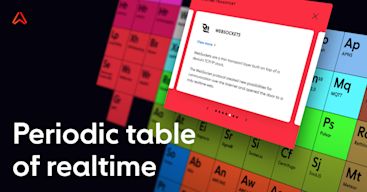
WebRTC
Learn about WebRTC, a free, open-source project enabling p2p comms in browsers and mobile apps via APIs, including audio, video, and data transfers.
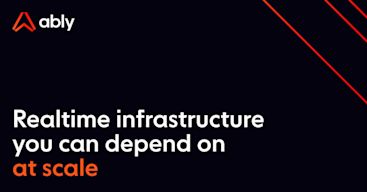
AWS SNS
SNS is a simple, distributed, cloud-native messaging service.
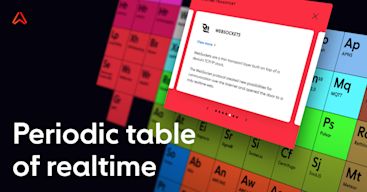
RethinkDB
Learn about RethinkDB, an open-source scalable JSON DB management system for realtime web applications that require continuously updated query results.