Send cross-platform, cross-device push notifications with our simple APIs.
Send notifications directly to users screens, from chat responses to news alerts, or game updates, whether or not an application is open and running.
Trusted by brands serious about realtime
Powers live chat, updates, analytics, and composition for millions of users.
Deliver live scores, updates and commentary to global fans with minimal latency on any device or browser.
Brings realtime seat updates for live event ticket buyers
Boost app engagement and user retention with targeted mobile notifications.
Velocity - Deliver cross-platform notifications (iOS, Android and web browsers) with a single request to our unified API.
Custom formats - Send custom notification formats and badges for iOS, Android, and web browsers
Efficiency - Maximize the ROI for your app with our efficiency boosting batching feature that reduces platform consumption.
Affordability - Make best use of your budget with per-minute, consumption-based pricing that’s built for scale and high user concurrency.
Performance - High volume throughput, powered by the largest WebSocket platform on earth providing 5+ years of 100% uptime.
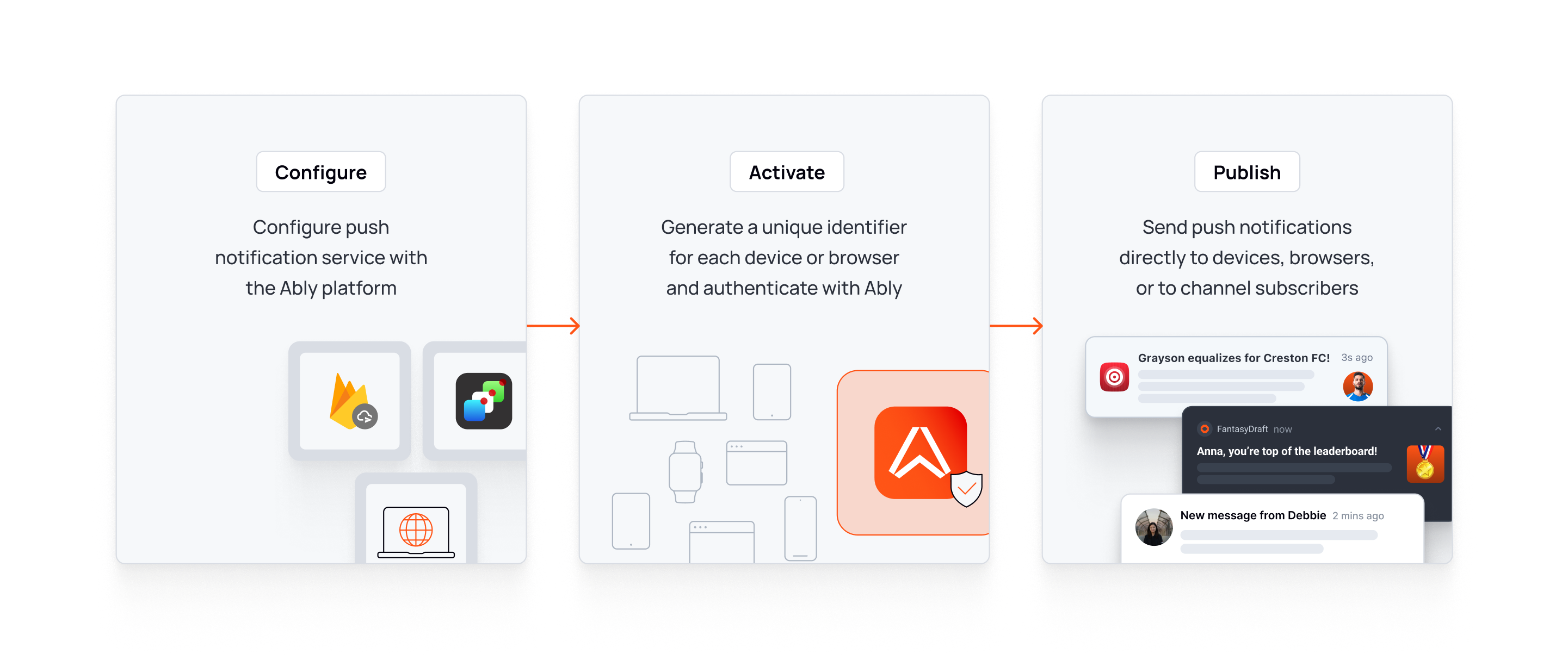
All the features you need to support push notifications
Ably sends push notifications to devices using Firebase Cloud Messaging or Apple Push Notification Service.
Ably sends push notifications to browsers using Web Push.
Sends push notifications to specific devices or browsers identified by unique identifiers, such as a deviceId or a clientId.
Messages are sent to channels to which multiple devices or browsers can subscribe.
Register devices or browsers, manage subscriptions, and send push notifications directly to devices, browsers or users identified by a client identifier.
Metachannels publish events and errors that aren’t otherwise available to clients.
Integrations, whatever your stack
From Webhooks to Lambdas to stream processors like Kafka, Ably has built integrations for a range of protocols, frameworks, databases and cloud services.
From Webhooks to Lambdas to stream processors like Kafka, Ably has built integrations for a range of protocols, frameworks, databases and cloud services.

Composable realtime experiences
Combine our products to create any experience you want. Your vision. Your approach.
Ably Chat: Deliver highly reliable chat experiences at scale
Ably Pub/Sub: Low-level APIs to build any realtime experiences at scale
Ably LiveObjects: Synchronized application state and live storage
Ably LiveSync: Sync database changes with frontend clients
Ably Spaces: Create multi-user environments
Ably Asset Tracking: Asset tracking across last mile logistics
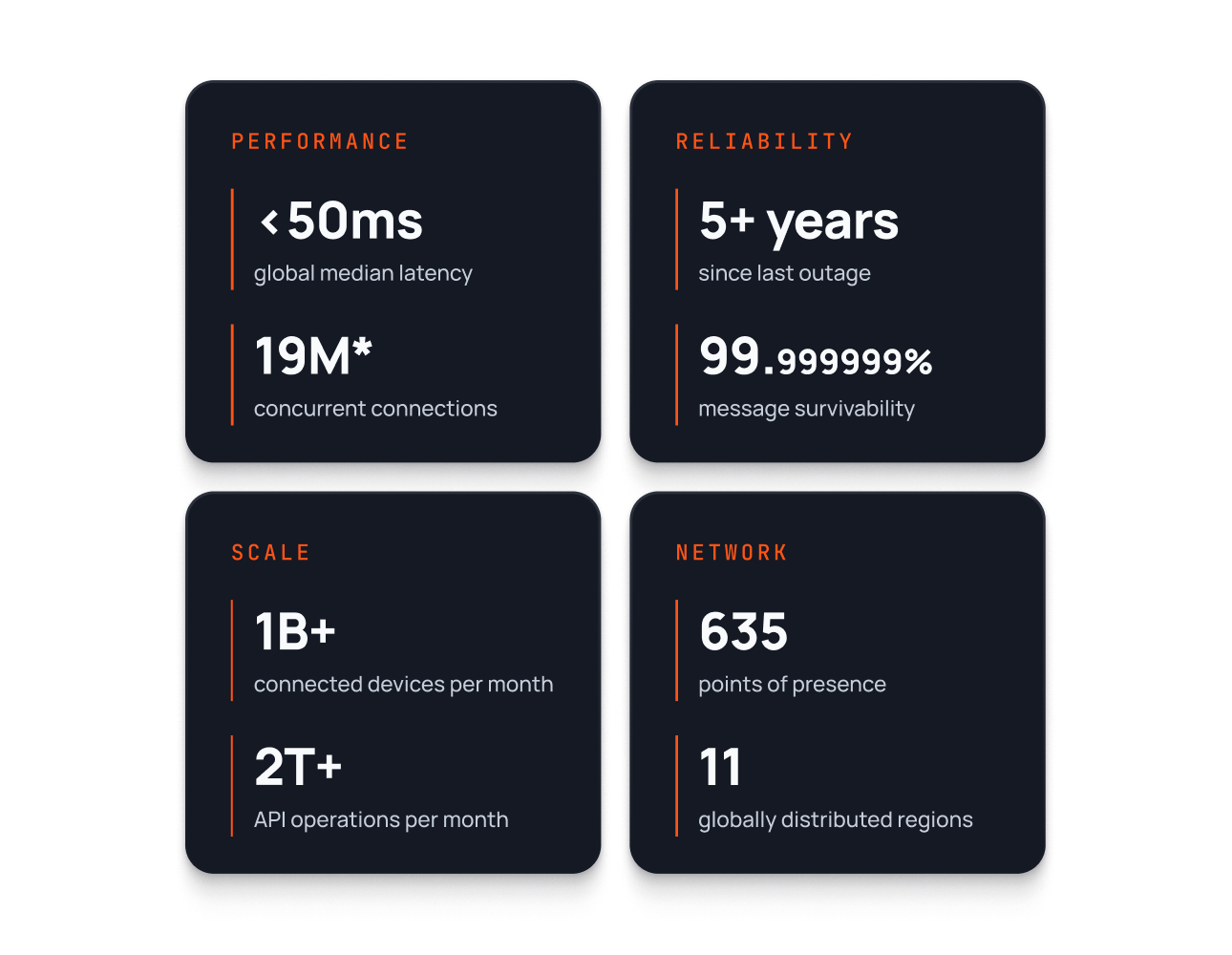
The definitive realtime experience platform of the internet
Performance - We focus on predictability of latencies to provide certainty in uncertain operating conditions.
Reliability - Designed to preserve continuity of service we ensure sufficient redundancy at a regional and global level.
Scale - Elastic and highly-available, Ably is built to handle extreme scale and high concurrent connections.
Network - Truly distributed global edge network. Delivers a globally consistent experience to users.

Ably is one of the key technologies that underpins our business. Its realtime experience infrastructure seamlessly supports HubSpot's entire realtime needs, helping us to meet our technical, business, and product development requirements. With Ably, we have innovated and taken new products to market much faster while significantly reducing our operational engineering overhead.
