Ably engineering
Filter by
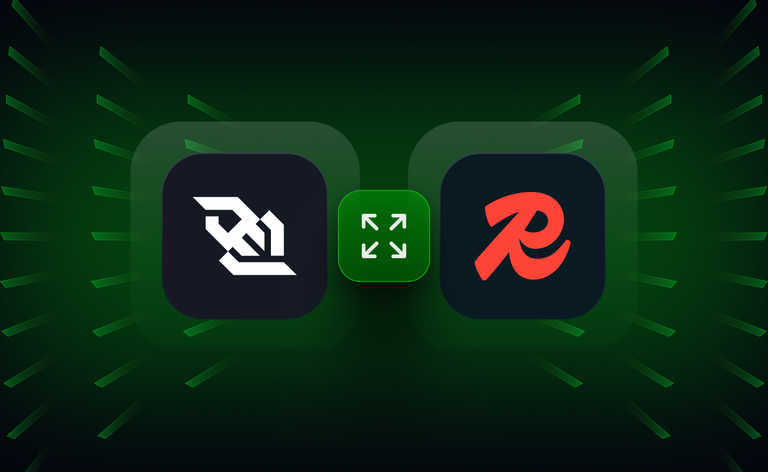
Ably engineering
19 min read
Scaling Pub/Sub with WebSockets and Redis
Dec 19, 2024
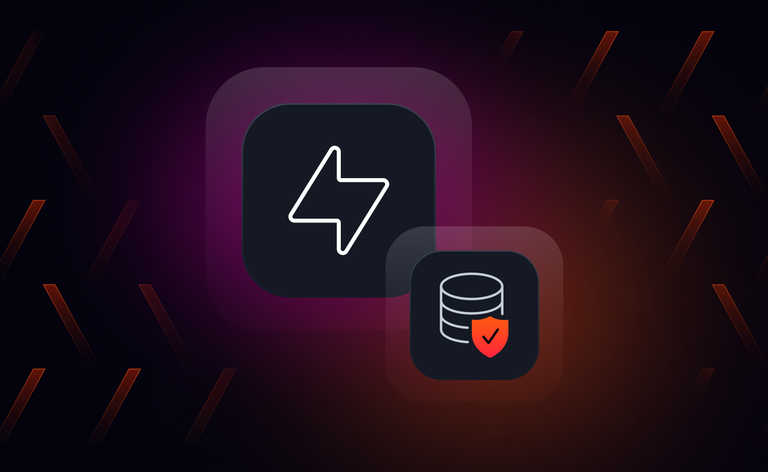
Ably engineering
6 min read
Data integrity in Ably Pub/Sub
Nov 21, 2024
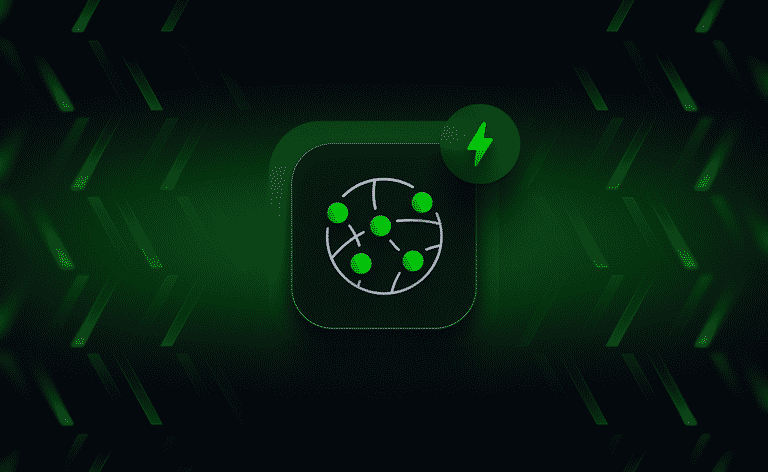
Ably engineering
12 min read
Optimizing global message transit latency: a journey through TCP configuration
Jul 29, 2024
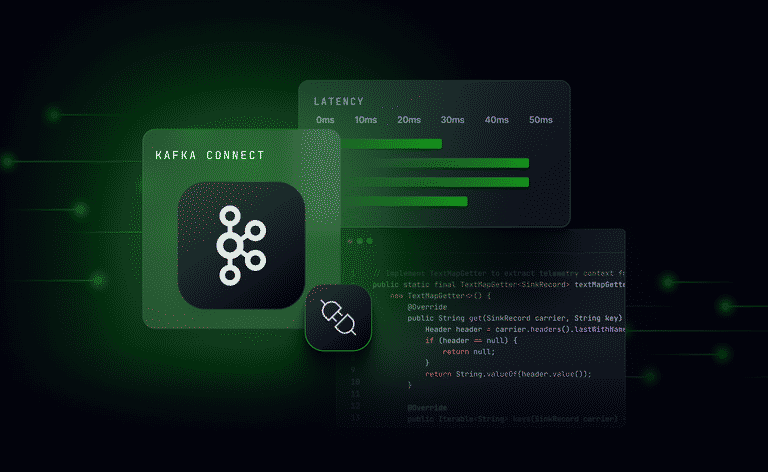
Ably engineering
10 min read
Measuring and minimizing latency in a Kafka Sink Connector
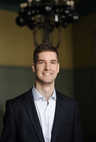
Jul 16, 2024
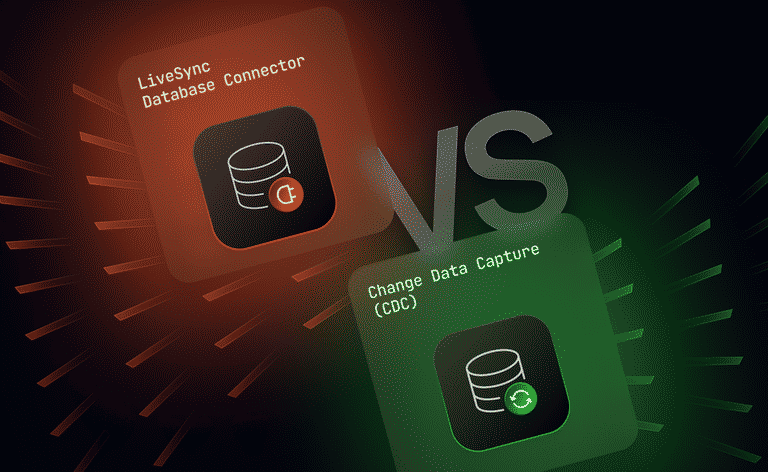
Ably engineering
6 min read
Database generated events: LiveSync’s database connector vs CDC
Jun 18, 2024
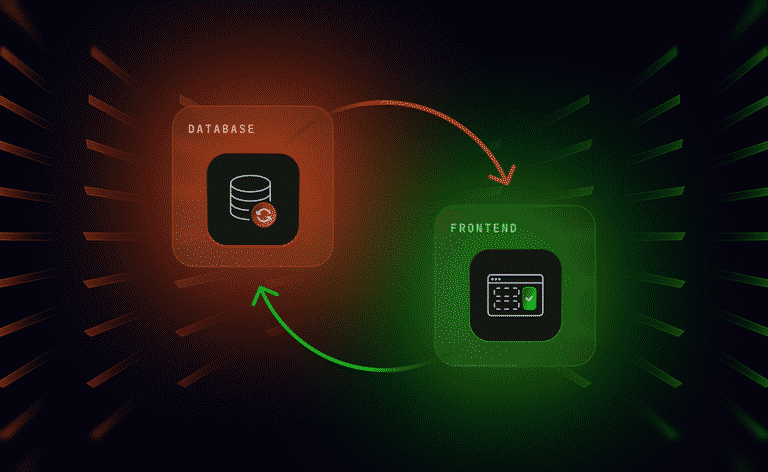
Ably engineering
15 min read
Reliably syncing database and frontend state: A realtime competitor analysis
May 15, 2024
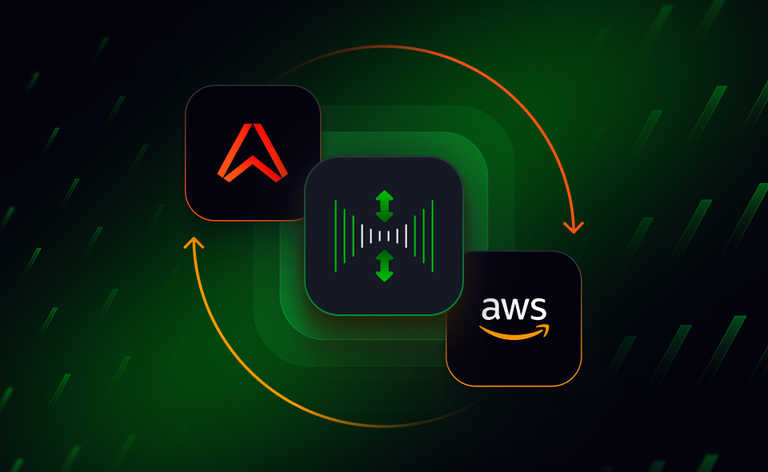
Ably engineering
7 min read
Overcoming scale challenges with AWS & CloudFront - 5 key takeaways
Apr 30, 2024
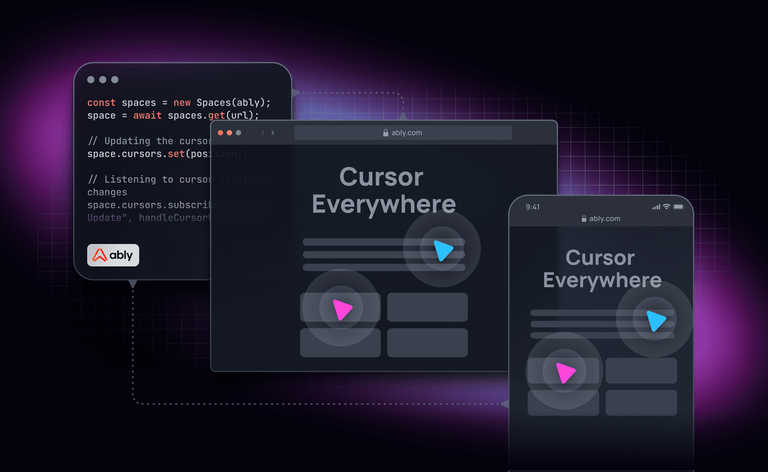
Ably engineeringCollaborative experiences
15 min read
Cursor Everywhere: An experiment on shared cursors for every website

Aug 18, 2023
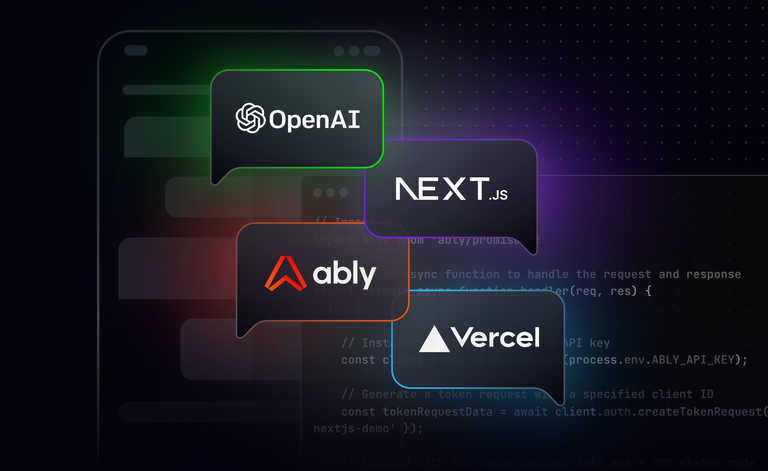
Ably engineering
24 min read
Group chat app with OpenAI's GPT

May 24, 2023
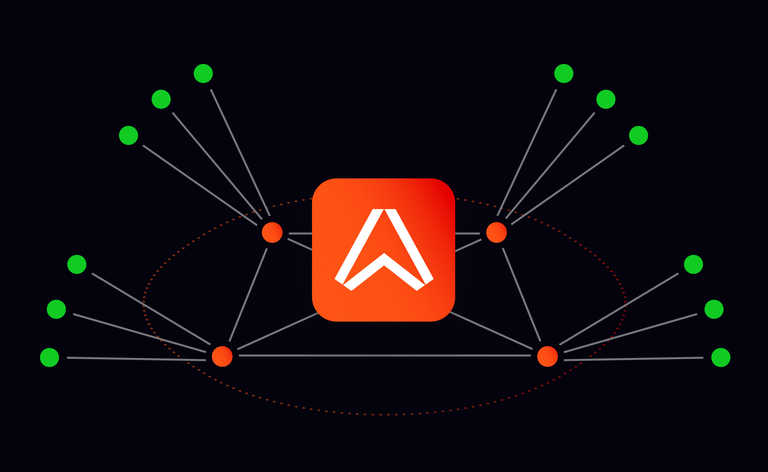
Ably engineering
14 min read
CRDTs are simpler and more common than you think

Feb 14, 2023
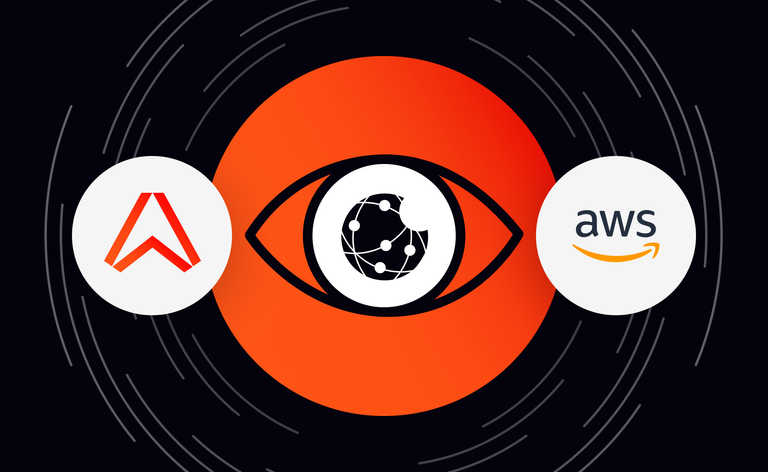
Ably engineering
14 min read
VPC peering vs Transit Gateway and beyond: Key choices in AWS network design
Sep 13, 2022
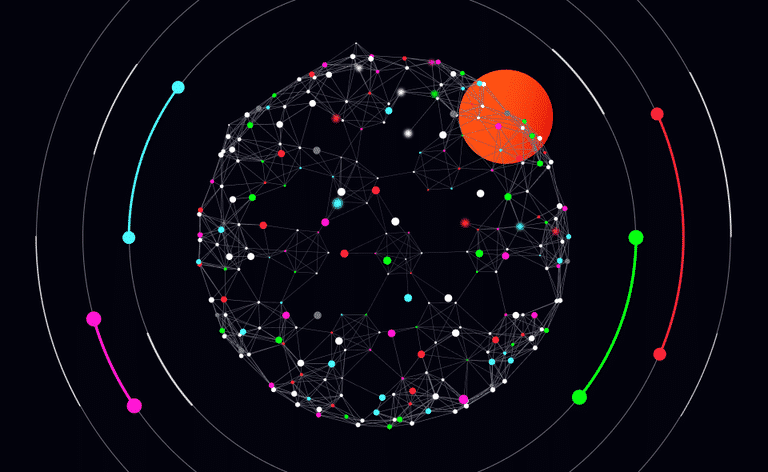
Ably engineering
8 min read
A globally-distributed architecture for reliable, low-latency edge messaging

Jun 16, 2022