Whether you’re connecting clients to servers, microservices to one another, or parts of your application to external services, you’re almost spoiled for choice when it comes to communication methods. There are, though, two that stand out: WebSocket and REST.
The good news is that, even though selecting the core technologies for your application is a serious responsibility, choosing between WebSocket and REST is relatively straightforward thanks to their different architectural choices, performance characteristics, and implementation considerations. That means each lends itself to solving problems where the other might struggle.
In this article, we’ll set out to clarify REST vs WebSocket, covering:
A refresher on what REST and WebSocket are
Their technical tradeoffs, including WebSocket vs REST performance
When you should use REST and when WebSocket is a better choice
Some of the gaps you’ll need to plug if you work with either protocol.
But if you’re looking for a quick summary, here’s what you need to know:
REST is ideal when you need easily scalable infrastructure for stateless CRUD operations and you want to stick with standard web tooling. For example, if you’re building an API to post updates to a social network
WebSocket is the right choice when you need realtime, bidirectional, low latency communication between client and server. For example, if you’re building a chat application.
What is REST?
Representational State Transfer (REST), is a set of principles that define one way to build APIs over HTTP. It’s easy to overlook, but REST isn’t a protocol, which means you have a great deal of flexibility to mold it to your needs. Its popularity is largely due to its simplicity and the fact that it reuses the methods and technologies already used for the web. And that largely defines the use cases where it does well and those where you’ll need to find an alternative.
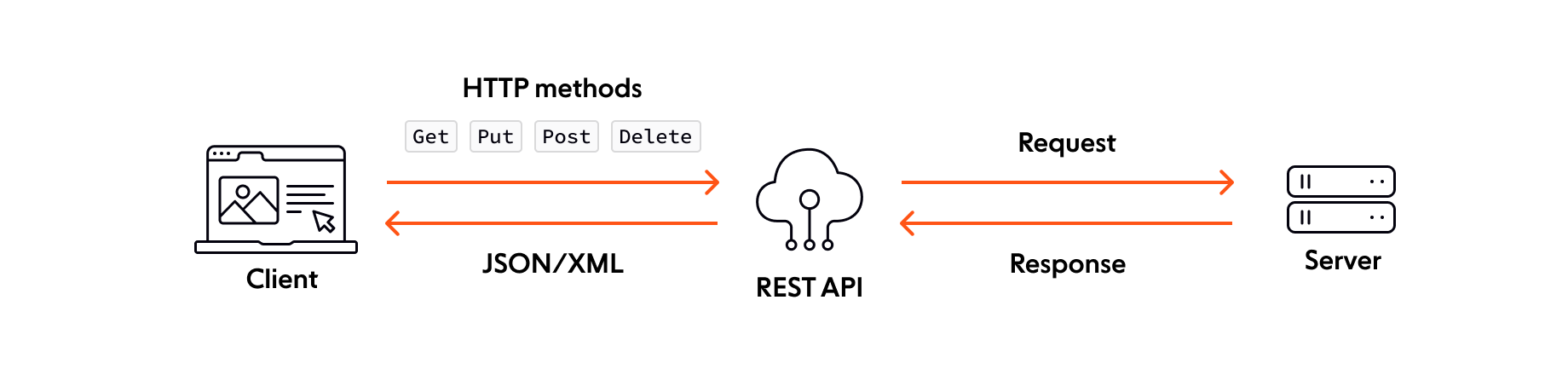
Let’s take a quick look at what sets REST apart from other approaches.
REST’s key characteristics
Stateless: Each request made to a REST API is independent and self-contained. That makes scaling much more straightforward. When traffic increases, you can add more servers behind a load balancer as there’s no need for a particular client to connect to the same server each time. Even though HTTP and REST are stateless, you can maintain long-running sessions by using client-side cookies to track session and log-in status.
CRUD: Each REST request uses the standard HTTP verbs (GET, POST, PUT, PATCH, and DELETE), which lines-up with Create, Read, Update, Delete operations.
Payload format: REST typically uses standardized message formats like JSON or XML for data exchange. But you can use whatever format of data you like, so long as you set the correct Content-Type header.
Cacheable: You can choose to allow clients, and intermediate infrastructure, to cache responses. That helps scaling and response times.
Synchronous: The HTTP request-response cycle that REST relies on is best suited to short-lived, straightforward transactions where a client waits for response before moving on to the next task.
What is WebSocket?
While REST works with short-lived, stateless communication, WebSocket provides an ongoing, low latency, two-way communication channel. That means the way you interact with WebSocket is different. So, rather than building a 'WebSocket API' as such, with specific endpoints, you open a connection and both sides can exchange messages as and when they need. This makes it ideal for realtime applications, such as chat, the live streaming of sports or financial data, and interactive, realtime collaborative environments like Figma, Miro, and Google Docs.
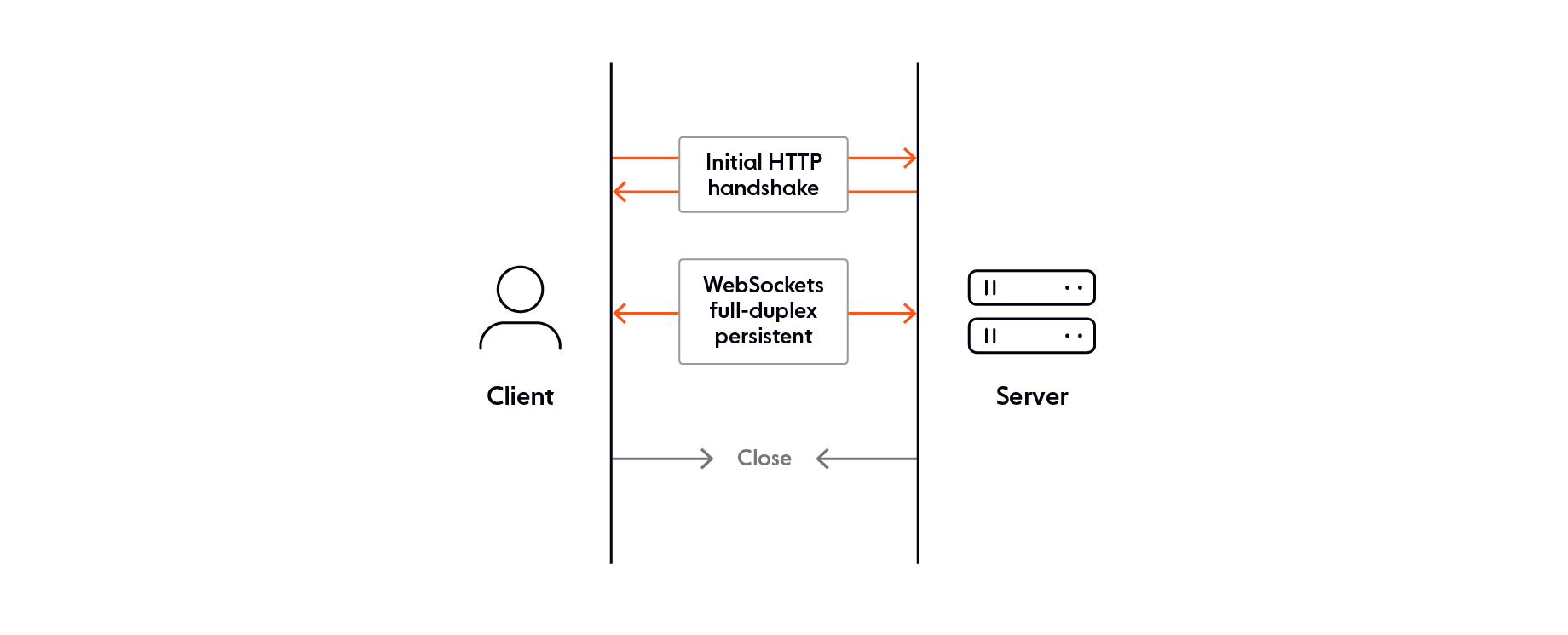
WebSocket key characteristics
Persistent connection: Each REST request requires a fresh HTTP connection, which increases latency due to the overhead of the HTTP handshake. WebSocket opens a connection once and then keeps it open for as long as you need it, reducing the time it takes to send a message.
Stateful: WebSocket’s persistent connection means that the client and server can keep track of what’s happened previously, rather than having to use cookies to track state, as with REST.
Full duplex communication: Both the client and the server can send data whenever they need and at the same time. In REST, only the client can initiate a new connection.
Payload format: WebSocket works with discrete messages, such as a JSON object or a Protobuf message. That’s in contrast with streaming media formats that send a constant flow of bytes. Importantly, WebSocket lets you send text or binary data and it’s up to you to handle serializing and deserializing that data.
Realtime: WebSocket minimizes latency wherever possible. A persistent connection helps, as do small headers (as low as two bytes).
WebSocket vs REST at a glance
As we’ve seen, the design of WebSocket and REST results in substantially different ways of connecting clients and servers. Let’s recap what sets them apart.
REST | WebSocket | |
Communication model | Request-response | Event-based |
Statefulness | Stateless | Stateful |
Scalability | Easy to scale | More complex to scale |
Data formats | Binary or text | Binary or text |
Overhead | Higher, as each interaction requires a new connection | Lower, after initial handshake |
Use cases | CRUD APIs, stateless transfer of discrete units of data | Realtime collaboration, chat, data broadcast, ongoing transfer of data |
Should I use WebSocket or REST?
Technical capabilities are good to know when considering the difference between WebSocket and REST. But things get really interesting when we look at the use cases. The question of whether you should choose WebSocket or REST comes down entirely to the problems you want to solve. And the likelihood is that you’ll end up with some mix of the two.
Let’s take a mobile banking application as an example. The bulk of its client-server communication, such as making a payment, is best suited to a REST API. The payee details, payment amount, and associated data fit the request-response model quite neatly.
But let’s say you also wanted to add live customer service chat to the banking app. For that functionality only, WebSocket would be the better choice. The persistent, stateful connection and low latency delivery make it a good match for interactive, realtime communication.
So, perhaps the question should be, “Can I use REST and WebSocket together?”. The answer is a firm “yes”. And, in fact, it’s quite common to use different communication methods within the same application depending on the problems at hand.
WebSocket vs REST use cases
To start thinking about where you should use REST and where you should use WebSocket, let’s compare their suitability for some common scenarios.
Use case | REST | WebSocket |
Chat | Inefficient as it would require either frequent manual polling or a workaround such as long polling. | Persistent, two-way connection with low latency suits WebSocket well to chat. |
Online gaming | Not suitable as the overhead of multiple request-response cycles will add too much latency. | A better match for realtime updates across multiple players participating in an online game. |
Data updates | Suitable for infrequent updates where immediate data refresh isn't critical. | Well suited to dashboards, sports data, and financial data where updates are time sensitive. |
Realtime collaboration | Not practical as latency is too high and REST lacks a way for the server to initiate message transfer, without workarounds. | Instant synchronization in both directions means changes from all participants are shared at the same time. |
Service integration | Well suited to integrating third-party services based around stateless interactions, such as payment processing. | Unlikely to be a good fit, as there’s less need for a persistent connection |
Public API | This is where REST excels, as it’s easy to deploy using standard web tooling. | Not a good fit as there’s no discovery mechanism for resources and it’s harder to scale. |
IoT device communication | Where devices need to send data at regular intervals or triggered by a state change, REST could be suitable. However, it limits communication to one-way and there are better options available. | A good fit for devices that need constant, two-way communication with the rest of the IoT network. |
Although it’s helpful to think on a use case by use case basis, there is a general rule that you can apply to decide where to use WebSocket and where to use REST. For realtime communication, use WebSocket. For request-driven interactions like typical CRUD operations, then REST is the better choice.
Can a REST API use WebSocket?
REST’s flexibility means that you can choose precisely how to implement your REST API. For example, HTTP’s latency compared to other protocols might frustrate you. Could you swap it for WebSocket, instead? In theory, you could. The SwaggerSocket open source project tried to do just that. But REST and HTTP go together well because they’re both designed around the request-response model. WebSocket’s stateful, event-based model means it’s not a one-for-one replacement. If the problem you want to solve calls for REST, then use REST.
Options for building REST and WebSocket infrastructure
Choosing between WebSocket and REST is really just the first step. Keep in mind, neither of them tells you much about the infrastructure you'll need to run them on. For REST, building scalable HTTP backends is pretty much a solved problem. But that’s not the case for WebSocket. Persistent, stateful connections increase the load on the server and complicate scaling.
Thankfully, there’s a broad variety of libraries, frameworks, and hosted services available to simplify building and managing your application’s backend. They tend to fall into four categories:
Host your own backend: Although you’re taking on additional DevOps burden, you can host your own WebSocket backend with one of the following methods:
Pure WebSocket libraries: Most languages have an official or semi-official implementation of the WebSocket protocol. For example, 'ws' for NodeJS. They give you low-level WebSocket functionality that can be good if you just want a lightweight implementation or you really need to create a custom solution.
Fully-featured messaging libraries: One level higher are messaging libraries like SignalR for .NET or Socket.IO for NodeJS. They take care of more of the problem, such as automatic reconnection.
Use a framework: Spring Boot for Java also supports WebSocket natively.
Use a specialized hosted service: Depending on your use case, there might be a software as a service offering that offers a specialized WebSocket backend. For example, chat SaaS APIs are common. However, you’ll need to be comfortable with the design choices the vendor makes.
Use a backend as a service (BaaS): Platforms including Firebase and Supabase offer limited realtime functionality built on top of WebSocket. However, scalability and message guarantees are often lacking.
Use a realtime platform as a service (PaaS): For applications where reliability, data integrity, and scalability are important, you should consider using a dedicated realtime PaaS. Ably’s realtime PaaS helps you get to market faster, gives you global managed infrastructure, and offers rich functionality in addition to pure WebSocket.
With Ably, your application can take advantage of:
A global edge network: Wherever your app’s users are in the world, Ably edge locations ensure <50 ms latencies right across our network.
Not only WebSocket: Ably selects the right protocol for your application’s needs and network conditions, choosing from options such as WebSocket, Server Sent Events, and MQTT.
Resilient delivery: Ably guarantees that messages arrive in order and on time.
99.999% uptime: Ably’s network routes around failure to ensure your application keeps on delivering.
Scale to meet demand: As demand grows, Ably scales from thousands to billions of messages.
Great developer experience whatever your tech stack: With SDKs for more than 25 languages and frameworks, integrations with common tooling, and industry leading documentation, Ably gives you the tools to become productive quickly.
Sign up for your free Ably account and start building today.
Recommended Articles
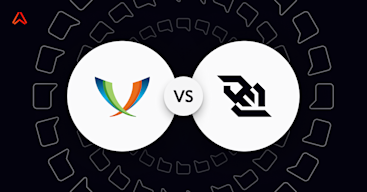
XMPP vs WebSocket: Which is best for chat apps?
Learn about the features of the XMPP and WebSocket protocols - and which is best for chat apps based on their pros and cons - in our comparison guide.
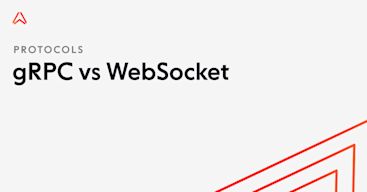
gRPC vs. WebSocket: Key differences and which to use
Discover the different features, performance characteristics, and use cases for gRPC and WebSockets to help understand which technology suits your needs best.
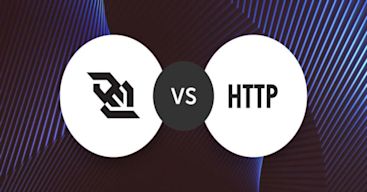
WebSockets vs HTTP: Which to choose for your project in 2024
An overview of the HTTP and WebSocket protocols, including their pros and cons, and the best use cases for each protocol.