This article explores the differences between two popular realtime web communication technologies: gRPC and WebSocket. Both support bidirectional full-duplex communication and allow the server to push messages to the client without polling from the client side. However, beyond some similarities, gRPC and WebSocket have plenty of differences, so the choice between them depends on exactly what you need for your project.
What is gRPC?
gRPC is an open source Remote Procedure Call (RPC) framework initially developed at Google in 2016 with two goals in mind:
Create a high throughput alternative to HTTP JSON APIs
Additionally, enable bidirectional streaming, replacing the need for sockets in some circumstances
To accomplish this, gRPC uses HTTP/2 as the transport protocol and Protobuf as the wire format.
In addition to these functional goals, gRPC provides a productive developer experience by offering a framework to predefine the procedures (functions) that can be called remotely, and the structure of the data those procedures can accept or return.
By default, gRPC uses Protocol Buffers (Protobuf) Interface Definition Language (IDL) to define the schema. Here’s an example of what it looks like:
// The greeter service definition.
service Greeter {
// Sends a greeting
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
// The request message containing the user's name.
message HelloRequest {
string name = 1;
}
// The response message containing the greetings
message HelloReply {
string message = 1;
}
It’s up to the server to implement this interface, then the client can call it transparently over the network with only a small latency.
At this point you might be wondering, which client libraries does gRPC support? All of them, pretty much! Here’s how it works.
After you define your interface like in the snippet above, Protobuf can generate idiomatic client code (stubs) that can call the remote procedures in almost any programming language.
This is a big draw for gRPC and Protobuf in general because it means programs written in different languages can call each other’s procedures. For example, a Go microservice can send logs to a Java microservice, or a Ruby server can stream data to an Android or iOS client.
What about on the web where WebSockets reign supreme?
Unfortunately, it’s not the best news.
While gRPC does work in the browser with gRPC-web, request streaming (client streaming) and bidirectional streaming are not supported due to their dependence on HTTP/2.
What is gRPC-web?
As you will recall from the introduction, gRPC relies on HTTP/2 under the hood.
Unfortunately, while modern web browsers do actually support HTTP/2, they don’t give client library developers the fine-grain control they need to implement the gRPC specification.
To work around this, gRPC-web requires a small proxy on the server to translate the requests and responses from HTTP/2 to HTTP/1.
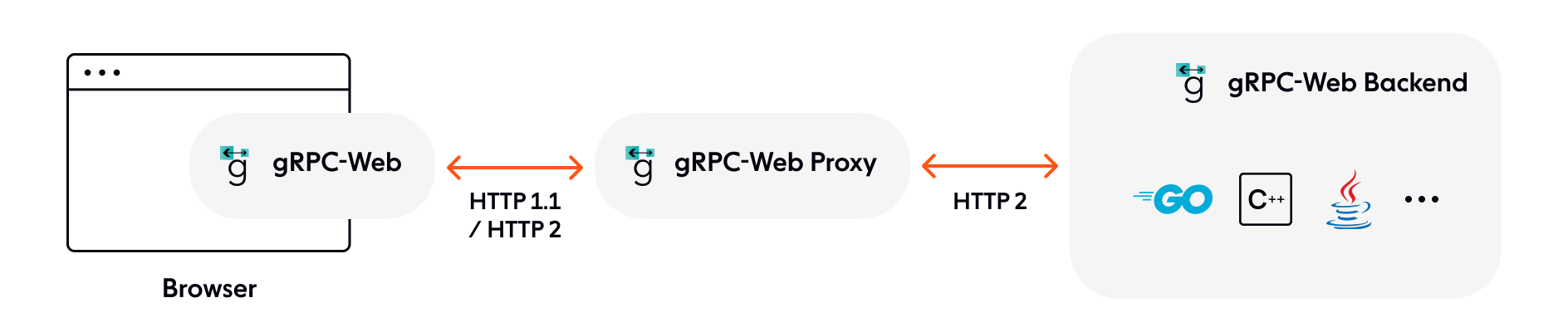
This is good for interoperability, but since the transport protocol is downgraded from HTTP/1 to HTTP/2, we lose some features and benefits. Most notably for our comparison with WebSockets, request streaming and bidirectional streaming.
This makes gRPC-web inappropriate for many bidirectional use cases like speech recognition or chat, at least for now.
gRPC-web plans to implement request streaming and, in turn, bidirectional streaming using Web Transport by “2023+”. With that said, extending gRPC-web’s streaming capabilities has been the subject of much debate among users since 2016, and it remains unclear when it will be fully available.
gRPC strengths
Let’s recap some of the advantages of gRPC, plus some others we think you should know about:
Cross platform: Libraries are available in several languages, and the Protobuf format allows them to interoperate smoothly (so you can easily use clients and servers written in different languages).
Multiple parallel requests: When used with HTTP/2, gRPC supports multiplexing many requests on one connection, allowing for higher throughput.
Bidirectional streaming: When used with HTTP/2, gRPC supports bidirectional streaming, which sometimes replaces the need for sockets.
Lightweight messages: Compared to JSON or XML, which is text-based, Protobuf uses a compact binary format.
Optional compression: Because messages are lightweight by nature, you might not need compression, which can save valuable CPU resources on mobile and IoT devices.
High performance encoding: Protobuf is 5x faster than JSON at encoding messages, which will be tangible in high throughput situations.
Strongly typed message structure: By predefining the shape of messages with Protobuf, developers are less likely to make errors like forgetting a required field. As a bonus, defining schemas with Protobuf allows for versioning.
gRPC weaknesses
For all the benefits gRPC affords, it’s not without its limitations. Here’s a quick recap, as well as some other weaknesses you might want to be aware of:
Limited browser support: This is the big one when comparing gRPC and WebSockets. gRPC-web does not support request streaming, bidirectional streaming, or multiplexing because of its reliance on HTTP/2.
Difficult to debug: Protobuf messages aren’t human-readable compared to JSON or XML. To decode a Protobuf message, you need the original schema to hand. This can break tools like network traffic inspectors. For instance, Lyft ran into this exact situation and had to build a new tool.
Lack of maturity: Compared to WebSockets, which have 27K questions on StackOverflow, gRPC currently has little over 6K. While gRPC has been adopted by big companies we all know and respect like Lyft and Uber, it’s not too widespread.
Complexity: gRPC can be difficult to set up because it relies on HTTP/2 and Protobuf. It might be too much for smaller apps, and may need extra work to deploy well in a distributed system.
Header weight: Although headers are compressed with HTTP/2 using HPACK, they add a small overhead to each request compared to WebSockets.
What is WebSocket?
The WebSocket technology is a W3C standard that was introduced in 2008. A WebSocket connection is initiated by the client using an HTTP/1.1 request that gets "upgraded" by the server to a full-duplex, bidirectional communication channel. This connection persists until it is closed explicitly by either the client or the server, and, unlike HTTP, it imposes very little data transmission overhead. WebSocket is in widespread use by many companies, including HubSpot, Figma, and DAZN, to name just a few.
WebSocket can transmit both text (string) and binary data. The WebSocket protocol allows you to specify a subprotocol during the initial HTTP/1.1 handshake that can be used on top of WebSockets (for example, MQTT). Alternatively, you can define your own protocol on top of raw WebSockets if, say, you need custom data semantics or extra features such as publish/subscribe messaging.
WebSocket strengths
Here are some of the key strengths of WebSockets:
Cross platform: There are numerous libraries and frameworks implementing the WebSocket protocol across all programming languages and development platforms.
Built for the web: Browsers natively support the WebSocket API, which is an advantage compared to gRPC.
Minimal transmission overhead: Unlike earlier HTTP-based approaches (such as long polling), the WebSocket protocol uses persistent connections rather than a continuous request/response cycle. When it comes to realtime communication, WebSockets require less bandwidth and provide lower latency compared to HTTP, reducing the load on both the client and the server.
Supports text and binary: You can use any text or binary data format for the data. You could choose a text format to simplify debugging or a compact binary format to maximize efficiency.
Bidirectional streaming: As an event-driven technology, WebSocket allows data to be transferred without the client requesting it. This characteristic is desirable in scenarios where the client needs to react quickly to an event (especially ones it cannot predict, such as a fraud alert).
WebSocket weaknesses
While WebSockets have many advantages, they also suffer from some drawbacks:
Challenging to scale: The WebSocket protocol is stateful. This can be tricky to handle, especially at scale, because it requires the server layer to keep track of each individual WebSocket connection and maintain state information.
Reliability issues: WebSockets don’t automatically recover when connections are terminated – this is something you need to implement yourself, and is part of the reason why there are many WebSocket client-side libraries in existence.
Firewall problems: Certain environments (such as corporate networks with proxy servers) will block WebSocket connections.
No message structure: The event object's lack of structure makes it easy to keep adding more data to the event, causing it to become bloated. Consequently, your endpoints can lose their sense of responsibility and become unwieldy. Additionally, its dynamic nature makes it easy to forget or add fields by mistake.
No multiple parallel requests: You can use multiplexing with WebSocket to improve performance, but you must either use a third-party library or support it in your code. Both options add complexity to your project.
What are the differences between gRPC and WebSocket?
gRPC and WebSockets both fundamentally enable sending and streaming data, but that doesn't mean they don’t also have their differences.
gRPC is a communication framework that uses Protobuf as the wire format and HTTP/2 as the transport protocol. It’s typically used to enable server-server communication, but is also applicable in the last mile of distributed computing to connect devices, mobile applications, and browsers to back-end services. For example, Lyft uses gRPC to enable microservices implemented in different programming languages to communicate using the same semantic language, then stream the driver’s location to their Android and iOS apps.
WebSockets, on the other hand, is an application protocol specifically designed to exchange data in real time between browser and server. It’s frequently adopted on Android and iOS as well, but developers don’t typically reach for WebSockets when they need to send or stream data between servers.
It’s worth reiterating that gRPC is a framework built on the HTTP/2 protocol, whereas WebSockets is “just” a protocol.
In some ways, it would be fairer to compare WebSockets to HTTP/2 although, as you will now know, gRPC-web cannot use HTTP/2 and downgrades the protocol to HTTP/1, where request streaming and bidirectional streaming aren’t supported. This usually makes WebSockets a more attractive option for any project with a web client.
Now that we have discussed the differences between these technologies, let’s compare them in one tidy table.
Mode | gRPC | gRPC-Web | WebSockets |
Unary | Y | Y | Y |
Response streaming (server streaming) | Y with HTTP/2 and Server Sent Events | Y with HTTP/1 and XHR streaming | Y |
Request streaming (client streaming) | Y | N | Y |
bidirectional Streaming | Y | N | Y |
gRPC vs. WebSocket performance
Neither option is inherently more performant than the other. It depends on the specific needs and constraints of your situation.
However, if you need to send or stream large amounts of data, gRPC is likely to perform well due to its ability to process multiple requests in parallel and use the quick and lightweight Protobuf format. According to DreamFactory, gRPC connections can perform 10x faster than HTTP API connections.
When it comes to real time, WebSockets may be more efficient than gRPC because it uses a persistent TCP connection that is normally only closed only when communication is finished. Additionally, WebSockets keeps header overhead to a minimum, only requiring them for the initial HTTP/1 handshake. By comparison, gRPC still incurs some latency from headers and reconnecting after periods of inactivity.
gRPC vs. WebSocket data format
With gRPC, the .proto file defines and documents the binary data format explicitly. The binary format is compact, and you can tailor it very precisely to suit your needs. Some effort is required to design it, but this might be worthwhile if the data or infrastructure is complicated (for example, if you have existing components written in different programming languages).
WebSocket has no “official” format specification - it can use any binary or text format. You can use standard options like JSON, Protobuf, and MessagePack, or create your own custom format if necessary.
gRPC vs. WebSocket benchmark
Unfortunately, there aren’t any public benchmarks comparing gRPC and WebSockets directly. There are two likely reasons for this.
First, gRPC is a framework, while WebSockets is a protocol. It might make more sense to compare WebSockets with the underlying protocol used by gRPC which is HTTP/2, or HTTP/1 in the case of gRPC-web.
Second, although gRPC and WebSocket capabilities overlap, they are normally adopted for fundamentally different purposes. gRPC is typically used to enable server-server communication with high throughput (for example, streaming logs between microservices). By comparison, WebSockets are designed first and foremost for web browsers, so you wouldn’t necessarily consider them to enable server-server communication in the first place.
Understanding the characteristics of gRPC and WebSockets above can help you determine which is best for your situation, but running your own benchmark is the only way to accurately measure their performance based on your specific needs. This allows you to adjust variables such as batch size, compression configuration, concurrent connections, and target latency, and see the impact on events per second (EPS) and CPU utilization.
gRPC vs. WebSocket security
gRPC supports Transport Layer Security (TLS) for encryption and authentication. Note that you can use Google's ALTS variant of TLS (when the app is running on the Google Cloud Platform). You can also use token-based authentication (such as OAuth2) as an extra level of security for your gRPC application.
With WebSocket, you can use TLS for encryption via the wss: URL scheme (similar to https). WebSocket doesn't impose any particular authentication method; you can use any of the methods that are typically available for HTTP, such as cookies, HTTP authentication, or TLS authentication. You can also implement your own mechanism (such as token-based authentication) if this suits your needs better.
gRPC vs. WebSocket scalability
WebSocket is a stateful protocol, which relies on both sides (but especially the server) storing information about the connection state and history of previous messages. You need more complex code to keep track of the state; additionally, the client can only communicate with a server process that has access to the current state. This can make it hard to scale out to a large number of WebSocket connections (for example, load balancing between servers is tricky). That’s not to say you can’t scale to handle millions of concurrent WebSocket connections; it’s just incredibly difficult to do it right.
HTTP ( used by gRPC) is stateless, meaning that any information needed to maintain communication gets retransmitted with each request/response exchange. Although this involves inefficient repetition of data in the headers, it also ensures that server processes are interchangeable, since none of them needs to remember anything about the clients they are communicating with. Consequently, scaling up to large numbers of connections is somewhat less complex than scaling WebSockets. However, as WebSockets are generally less resource-intensive than HTTP and more efficient at transmitting data, scaling a gRPC system requires more computing power and bandwidth compared to scaling a WebSocket-based system of similar size.
Should I use gRPC or WebSocket?
Whether you should use gRPC or WebSockets depends on the specifics of your use case. Below, we list the most common scenarios where gRPC / WebSocket is a strong choice.
When to use gRPC
Connecting polyglot services in a microservices-style architecture.
Connecting client devices (e.g., mobile devices) to backend services (ideally use cases that don’t involve high-frequency data updates).
Connecting point-to-point realtime services that need to handle streaming requests or responses.
When to use WebSocket
Realtime updates, where the communication is unidirectional, and the server is streaming low-latency (and often frequent) updates to the client. Think of live score updates, newsfeeds, alerts, and notifications, to name just a few use cases.
bidirectional communication, where both the client and the server send and receive messages. Examples include chat, virtual events, and virtual classrooms (the last two usually involve features like live polls, quizzes, and Q&As). WebSockets can also be used to underpin multi-user for remote procedure calls between client and server applications.synchronized collaboration functionality, such as multiple people editing the same document simultaneously.
Fanning out (broadcasting) the same message to multiple users at once. Various WebSocket libraries implement broadcasting over WebSockets (usually via pub/sub messaging). In contrast, gRPC does not natively support broadcasting.
Ably: An alternative solution for realtime experiences
As you can now see, both gRPC and WebSockets have their limitations when it comes to adding real time functionality to mobile and web applications.
While gRPC is a powerful framework, it's not a good fit for web browsers due to its dependence on HTTP/2. Although you can use a proxy to enable server streaming on the web, request streaming and bidirectional streaming are still not possible.
That makes WebSockets look like an attractive option for web clients until you remember they require additional work to achieve the delivery and scaling guarantees necessary to deliver a seamless real time experience in production. Scaling WebSockets is non-trivial because of their stateful nature - in addition to configuring a load balancer, you would need to write additional code to keep track of each individual connection and maintain state information across servers. What’s more, since WebSockets is “just” a protocol, there’s a lot of work needed to ensure messages arrive exactly once and in the correct order, even in the face of network issues.
Enter Ably.
Ably is a realtime experience infrastructure, and an alternative to using raw WebSockets. Our APIs and SDKs help developers build and deliver realtime experiences without having to worry about maintaining and scaling messy WebSocket infrastructure.
Key Ably features and capabilities:
Pub/sub messaging over serverless WebSockets, with rich features such as message delta compression, automatic reconnections with continuity, user presence, message history, and message interactions.
A globally-distributed network of datacenters and edge acceleration points-of-presence.
Guaranteed message ordering and delivery.
Global fault tolerance and a 99.999% uptime SLA.
< 65ms round-trip latency (P99).
Dynamic elasticity, so we can quickly scale to handle any demand (billions of WebSocket messages sent to millions of pub/sub channels and WebSocket connections).
Explore our documentation to find out more and get started with a free Ably account
Recommended Articles
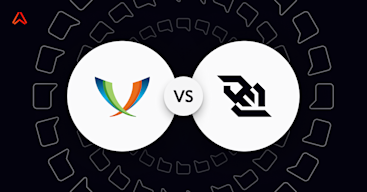
XMPP vs WebSocket: Which is best for chat apps?
Learn about the features of the XMPP and WebSocket protocols - and which is best for chat apps based on their pros and cons - in our comparison guide.
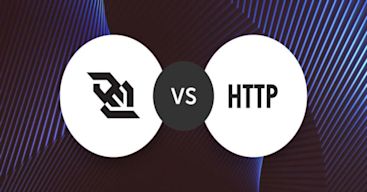
WebSockets vs HTTP: Which to choose for your project in 2024
An overview of the HTTP and WebSocket protocols, including their pros and cons, and the best use cases for each protocol.
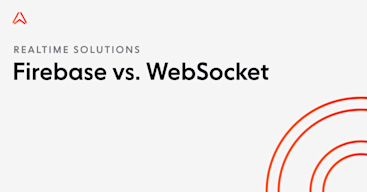
Firebase vs WebSocket: Differences and how they work together
We compare Firebase and WebSocket, two popular realtime technologies. Discover their advantages and disadvantages, use cases, and how they work together.