Introduction: Socket.IO and WebSocket aren’t apples-to-apples
WebSocket is a browser-native protocol for full-duplex communication over a single TCP connection. Socket.IO is a JavaScript library that builds on top of WebSocket and fallback transports. Though often compared, they serve different purposes and offer distinct tradeoffs when you're building large-scale, low-latency realtime systems.
TL;DR: WebSocket = protocol. Socket.IO = abstraction layer + feature set over multiple transports (including WebSocket).
What is WebSocket?
WebSocket is a low-level protocol defined by RFC 6455, enabling two-way communication between client and server over a single, long-lived connection. It's supported natively in most browsers and platforms, making it a foundational building block for realtime systems.
Pros:
Minimal latency
High throughput
Broad interoperability (browsers, servers, devices)
Standardized protocol
Cons:
Manual connection handling (no automatic reconnections)
No built-in reconnection logic or pub/sub abstraction (it's just a protocol)
No message guarantees
Scaling can be hard and requires additional logic
What is Socket.IO?
Socket.IO is a JavaScript library for realtime web applications, consisting of a Node.js server and browser client. It uses WebSocket as a transport when available, but can fall back to other methods like HTTP long polling. It provides developer-friendly features out of the box:
Pros:
Automatic reconnection
Event-based messaging
Broadcasting and room management
Middleware support (server-side)
Cons:
Higher message overhead
Custom protocol: not compatible with raw WebSocket clients
Single region
Weak message guarantees (defaults to 'at most once' delivery)
Less performant under scale
JS-focused ecosystem (limited support outside Node.js/JS)
You still need to build (and maintain) the backend infrastructure
WebSocket vs Socket.IO: Side-by-side comparison
Feature | WebSocket | Socket.IO |
---|---|---|
Type | Protocol | Library (with custom protocol layer) |
Transport | WebSocket only | WebSocket + fallbacks (HTTP long polling, etc.) |
Performance | High performance with minimal overhead | Higher overhead due to abstraction and custom framing |
Reconnection | Manual implementation required | Built-in automatic reconnection |
Messaging Format | Binary, text | JSON-based events |
Interoperability | Broad (native support across platforms and languages) | Limited (requires Socket.IO-compatible clients) |
Developer Experience | Requires boilerplate; more flexibility and control | Easier to adopt for Node.js developers; feature-rich |
Customizability | Highly customizable with protocol-level control | Lower customizability; built-in abstractions simplify setup |
Ease of Adoption | Steeper learning curve | Fast to prototype with; event-driven APIs |
Reliability | Depends on implementation | Reconnection and buffering built-in, but adds complexity |
Guarantees | No built-in delivery guarantees | No guarantees; requires custom handling |
Scalability | Complex without tooling or third-party services | Node.js-specific clustering; not designed for global scale |
Performance at scale: What to expect
When building large-scale realtime systems, performance differences between Socket.IO and WebSocket become more pronounced. Here’s what to consider:
1. Latency: WebSocket typically provides lower latency since it's a raw, binary-capable protocol with minimal overhead. It's a better fit for time-sensitive data flows like trading platforms, multiplayer games, or telemetry.
2. Message Overhead: Socket.IO wraps data in a custom event-based structure. This simplifies development but increases message size and adds serialization/deserialization steps that can impact throughput at scale.
3. Reconnection Logic: Socket.IO includes automatic reconnection with exponential backoff and state recovery features. While this is convenient, it introduces complexity and can strain backend resources under mass reconnection scenarios (e.g. after a network outage).
4. Compatibility and Lock-in: WebSocket is a standard protocol supported across platforms. Socket.IO requires a client that understands its proprietary framing, making it harder to mix tech stacks or integrate with non-JS systems.
In systems with thousands or millions of concurrent users, even minor increases in message size, latency, or reconnection load can impact performance and cost.
When to use each: Decision guide
Choose WebSocket if you need:
Full control over protocol behavior
Maximum efficiency and minimal latency
Compatibility across diverse environments
Standards compliance
Choose Socket.IO if you want:
Faster prototyping in Node.js environments
Built-in features like rooms and retries
A simplified developer experience
Acceptable performance tradeoffs in exchange for convenience
Scaling beyond WebSocket or Socket.IO
Both tools are excellent starting points for building realtime applications, but they present significant limitations when it comes to scaling reliably and globally. At high volumes, the demands of connection management, data delivery, and infrastructure resilience become difficult to handle manually.
Here are some of the key capabilities you’ll need to go beyond:
1. Elastic scalability across regions: Neither WebSocket nor Socket.IO provide built-in support for multi-region failover or automatic horizontal scaling. Building this manually involves considerable DevOps effort.
2. Advanced messaging guarantees: Features like message ordering, delivery guarantees (at-least-once, exactly-once), and idempotency are not natively handled by either tool. You’ll need to engineer this into your stack.
3. Presence, history, and fan-out: Maintaining online/offline user state, storing message history, or broadcasting to millions of recipients requires custom implementations, caching, and storage layers.
4. Resilience to network failures: Handling reconnect storms, managing session continuity, and preventing message loss across unreliable networks are complex to manage without a robust infrastructure.
5. Observability and operational insights: At scale, you need realtime insights into connection state, message flows, and failures. Neither Socket.IO nor WebSocket provide this out of the box.
If your team is spending more time building and maintaining realtime infrastructure than delivering user-facing value, it may be time to explore managed solutions.
Alternatives to Socket.IO and WebSocket
Socket.IO and WebSocket are just one part of the realtime story. While they work for many use cases, they often fall short when it comes to reliability, delivery guarantees, and effortless scale. Here are some common alternatives:
Protocol-level options
MQTT: Lightweight and optimized for constrained networks - ideal for IoT, not for the browser.
WebRTC: Great for peer-to-peer audio/video, not general-purpose data.
Messaging libraries
SignalR: A .NET-native alternative to Socket.IO.
ActionCable: Built into Ruby on Rails, but limited in scale and features.
Platform-as-a-Service (PaaS)
Chat-specific or vertical platforms: Fast for chat, but may lack flexibility.
BaaS (e.g. Firebase, Supabase): Quick to start, but realtime features hit limits under scale.
Realtime PaaS (e.g. Ably): Designed to handle the hard parts - from message guarantees to elastic scaling - so you don’t have to.
Why Ably?
Ably is a realtime platform designed to handle global-scale pub/sub, presence, and data delivery. It:
Uses WebSocket natively
Offers protocol adapters (Socket.IO bridge available)
Guarantees ordering, delivery, and integrity
Provides 99.999% uptime SLA
Eliminates scaling and operational overhead
Explore Ably's platform:
Recommended Articles

Pusher vs WebSockets: Pros, cons and key differences
Discover how Pusher and WebSockets compare, including their pros and cons, use cases, key differences, and the available alternatives.
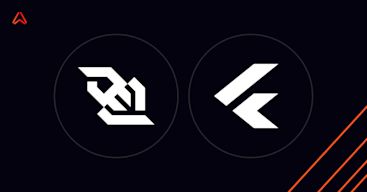
Building realtime apps with Flutter and WebSockets: client-side considerations
Learn about the many challenges of implementing a dependable client-side WebSocket solution for Flutter apps.
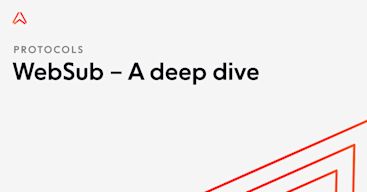
WebSub – A deep dive
This article explores the history of WebSub, how it works, use cases (including big names who have adopted it), and the challenges of using WebSub.