Welcome to the only guide you’ll ever need on SignalR vs WebSockets!
While both technologies enable realtime communication between apps, they each go about it in different ways, sometimes making it hard to feel confident about which is the right long-term choice for your project.
On this page, I’ll help you understand the most important differences and, by the end, you’ll know which is best-suited for your requirements.
The differences, at a glance
WebSockets is an unopinionated lower-level transport. It’s totally customizable, but if your goal is to implement realtime updates or build a realtime experience like chat or multiplayer collaboration, you might find yourself reinventing the wheel as you build out features every realtime app needs, like automatic reconnection and connection tracking.
SignalR, on the other hand, is a realtime communication technology from the Microsoft world. It’s available in a few different flavors, including a managed version on Azure (I’ll show you the different SignalR options and how they compare later on this page).
Fundamentally, SignalR builds on top of the WebSockets protocol to provide features you’d likely have to code yourself with raw WebSockets. While this is convenient, it comes at the expense of flexibility and possibly performance.
As we progress through the post, I’ll help you understand the nuanced differences so you can decide if the trade off between convenience and flexibility makes sense for you but, first, let’s cement our understanding of each technology.
What is WebSocket?
WebSocket is a communication protocol and web API that enables efficient two-way communication between apps.
They’re usually used in web apps (hence their name), but WebSockets also work on mobile, making them an attractive option for building cross-platform products with realtime features.
Compared to HTTP where the client initiates requests over a short-lived connection, WebSockets work by establishing a long-lived connection between the browser and the server, allowing either side to send fresh data as soon as it’s ready with minimum overhead.
This makes them a great choice for situations where two-way communication is needed such as chat and multiplayer collaboration.
Additionally, WebSockets are well-suited when you need to push frequent one-way updates from the server to the client - like live sports scores, the location of a courier on a map, fresh chart data, or perhaps a stock ticker for a new cryptocurrency.
Released in 2011, the WebSocket web API is supported in approximately 94% of browsers. The API looks something like this:
const ws = new WebSocket("ws://")
ws.onopen = () => {
console.log("WebSocket connection established")
}
ws.onclose =() => {
console.log("WebSocket connection closed")
}
ws.onmessage = event => {
console.log(`WebSocket message received: ${event.data}`)
}
ws.send("Hello, world!")
What is SignalR?
SignalR is a library that simplifies the process of adding realtime web functionality to applications.
It comes in several different flavors:
ASP .NET SignalR: The original library for ASP.NET developers. Note that this version is outdated (critical bugs are being fixed, but no new features are being added).
ASP .NET Core SignalR: The latest and most up-to-date version of SignalR for ASP.NET Core Developers.
Azure SignalR Service: The fully managed hosted version of SignalR.
SignalR uses WebSocket as the main underlying transport, while providing additional features, such as:
Automatic reconnections.
Fallback to other transports.
An API for creating server-to-client remote procedure calls (RPC).
The ability to send messages to all connected clients simultaneously, or to specific (groups of) clients.
SignalR uses hubs to communicate between clients and servers. A SignalR hub is a high-level pipeline that enables connected servers and clients to invoke methods on each other. On the client-side, it looks something like this:
const connection = new signalR.HubConnectionBuilder()
.withUrl("/chathub")
.build();
connection.on("ReceiveMessage", (user, message) => {
console.log(`${user} says ${message}`)
})
connection
.start()
.then( () => {
console.log("SignalR connection established");
}).catch(err => {
console.error(err.toString())
})
connection
.invoke("SendMessage", user, message)
.catch(err => {
console.error(err)
})
SignalR and WebSocket use cases
WebSocket use cases
We can broadly group WebSocket use cases into two distinct categories:
Realtime updates, where the communication is unidirectional, and the server is streaming low latency (and often frequent) updates to the client. Think of live score updates, GPS location tracking, live dashboards, or realtime alerts and notifications, to name just a few use cases.
Bidirectional communication, where both the client and the server send and receive messages. Examples include chat, virtual events, and virtual classrooms (the last two usually involve features like live polls, quizzes, and Q&As). WebSockets can also be used to underpin multi-user synchronized collaboration functionality, such as multiple people editing the same document simultaneously (think of Figma).
SignalR use cases
As SignalR is a WebSocket implementation, you can use it to engineer the same types of realtime features you’d build with raw WebSockets. Examples include:
Realtime dashboards.
Live chat.
Collaborative apps (e.g., whiteboards).
Realtime location updates.
Push notifications.
High-frequency updates (for use cases like realtime multiplayer gaming and streaming live score updates).
Comparing WebSockets and SignalR (comparison table)
Protocol | SignalR | WebSockets |
Compatibility (server) | Primarily associated with ASP.NET Core. | Can be implemented in various server-side technologies, including Node, Java, Python, etc. |
Compatibility (client) | Official client libraries available for JavaScript, C#, and Java (Android), but not Swift (iOS). | Can be implemented in various frontend technologies, including JavaScript, Java (Android), and Swift (iOS). |
Ease of use | Easier to use for developers as it abstracts away some of the complexities of handling different transport mechanisms. | Requires more low-level handling of the WebSocket protocol, which can be more challenging for some developers. |
Flexibility | Less flexibility and control | More flexibility and control |
Performance | Reliable, scalable
| Low latency, high throughput |
Scalability | Provides built-in support for scaling out to multiple servers using backplanes, making it suitable for large-scale applications. | While it can be used in a load-balanced environment, scaling WebSockets to a large number of connections may require additional considerations. |
Read on to learn more about how these two popular protocols compare in detail.
Compatibility
WebSocket compatibility
The WebSocket protocol is well-defined and implemented by most modern web browsers and servers. As a result, they are compatible with almost every technology stack.
The only time you might find WebSocket support to be lacking is when working with resource-constrained devices like IoT devices, but it’s increasingly rare, and there’s always fallbacks like long polling available, even though they’re less performant.
SignalR compatibility
SignalR has client libraries available for most platforms, with one notable exception for Swift, where there’s a library, but it’s not officially maintained.
Unfortunately, SignalR server compatibility is not so widespread.
SignalR on the server is tied to ASP.NET Core. If you’re already working with ASP.NET Core, no problem, but if you’re not, you can’t run SignalR on your own server. There is one option available, though. If you’re not using ASP.NET Core but you’re still keen on SignalR, you could use Azure’s hosted SignalR offering.
In a nutshell, Azure runs SignalR on their servers instead of yours. Now you don’t have to worry about server-compatibility – just point the same SignalR client at Azure, and watch the realtime messages roll.
Ease of use
WebSocket ease of use
WebSockets are a lower-level transport with a simple and minimal API. They are totally customizable, but therein lies the trade-off when it comes to ease of use.
WebSockets are versatile enough you can use them to build a completely custom messaging layer. The code can take the precise shape you imagine, while also allowing you fine-grain control over where to squeeze out performance versus where to make a compromise.
The downside with custom protocols is that they are, well, custom. As the developer, you need to code your own connection management, event handling, compression, message-routing logic (Pub/Sub is a popular pattern), and other services provided by libraries like SignalR.
SignalR ease of use
With SignalR, you get most of the features you need to build a typical real time messaging experience out-of-the-box. This doesn’t just make WebSockets easier to use, it’s much faster to get started as well, since you don’t have to reinvent the wheel.
Under the hood, SignalR manages the connection for you, and provides built-in features for serializing and deserializing messages using JSON or MessagePack protocols. SignalR also handles scenarios such as connection failure, reconnection, authentication, authorization, etc.
Flexibility
WebSocket flexibility
Because WebSockets is a lower-level protocol, it gives you total flexibility. You can decide how to manage the connection and decide what reconnection strategy to implement - for example, will you try to reconnect immediately or implement an exponential backoff strategy?
The shape and format of the realtime messages is entirely up to you as well. To give you an idea, SignalR does not guarantee message ordering. If this is important for your app, when you use WebSockets directly, you could attach a timestamp or sequence number to every message at the protocol level.
WebSockets also enables you to choose the message serialization format. While JSON is great for many applications, you also have the option to use a specialized format like MessagePack or Protobuf, depending on your requirements.
SignalR flexibility
While SignalR’s hub abstraction is plenty flexible for most use cases, it is inherently less flexible than WebSockets due to the fact it’s an abstraction.
With a library like SignalR, the aim is to provide a general solution that’s likely to work for the majority of use cases. While there are configuration options available (and you can always fork the code if you’re feeling ambitious), it might be that by the time you've adapted SignalR to your purposes, you may as well have used WebSockets directly for the inherent flexibility afforded because of their nature as a transport rather than a library and protocol on top.
Performance
WebSocket performance
Out of the box, WebSockets are as performant as they get. But, like we’ve explored in other sections on this page, there’s ordinarily features to add on top that will inevitably add overhead - how much or little, that is up to you.
SignalR performance
SignalR, on the other hand, is a higher-level library built on top of WebSockets and other transport technologies. While SignalR simplifies realtime communication and offers additional features like automatic reconnection, it may introduce some performance overhead due to its abstractions and features.
Scalability
WebSocket scalability
WebSockets have some unique scaling challenges due to their stateful nature.
With a protocol like HTTP, connections are short-lived and requests are self-isolated, making it easier to distribute requests across servers using a load balancer.
WebSockets, on the other hand, use a long-lived connection and it’s up to the server to manage the state associated with the long-lived connection. This introduces a challenge, especially when you introduce multiple servers, as you now need a backplane to synchronize state between servers.
SignalR scalability
Since SignalR uses WebSockets, it fundamentally suffers from the same problem. That being said, if you’re self-hosting SignalR, they offer a Redis add-on to simplify the process of adding a backplane.
To give you a sense of what it looks like, it looks something like this:
services.AddSignalR().AddStackExchangeRedis("<your_Redis_connection_string>");
However, expect to do a lot of additional configuration as you set up a load balancer and set out to test your SignalR server under real world conditions.
WebSocket pros and cons
WebSocket pros
Before WebSocket, HTTP techniques like AJAX long polling and Comet were the standard for building realtime apps. Compared to the HTTP protocol, WebSocket eliminates the need for a new connection with every request, drastically reducing the size of each message (no HTTP headers). This helps save bandwidth, improves latency, and makes WebSockets less taxing on the server side compared to HTTP.
Flexibility is ingrained into the design of the WebSocket technology, which allows for the implementation of application-level protocols and extensions for additional functionality (such as Pub/Sub messaging).
As an event-driven technology, WebSocket allows data to be transferred without the client requesting it. This characteristic is desirable in scenarios where the client needs to react quickly (instantly) to an event, as soon as it occurs.
Most programming languages support WebSockets, and there are numerous libraries and frameworks implementing the WebSocket protocol. Additionally, almost all modern browsers natively support the WebSocket API.
WebSocket cons
The WebSocket protocol is stateful. This can be tricky to handle, especially at scale, because it requires the server layer to keep track of each individual WebSocket connection and maintain state information.
WebSockets don’t automatically recover when connections are terminated – this is something you need to implement yourself, and is part of the reason why there are many WebSocket client-side libraries in existence.
Even though WebSockets generally benefit from widespread support, certain environments (such as corporate networks with proxy servers) will block WebSocket connections.
SignalR pros and cons
SignalR pros
Multiple backplane options to choose from when scaling out: Redis, SQL Server, or Azure Service Bus (note that there’s no need to configure and manage a backplane when using the fully managed version, Azure SignalR Service).
Part of the ASP. NET Framework, which makes it easy to use SignalR in combination with other ASP. NET features like authentication, authorization, and dependency injection.
SignalR uses WebSockets wherever possible, but it also supports two fallback transports: Server-Sent Events, and HTTP long polling, for environments where the WebSocket transport is blocked/unsupported.
SignalR cons
SignalR offers rather weak messaging quality of service QoS - ordering and delivery are not guaranteed. You’d have to develop your own mechanism to ensure robust messaging (e.g., adding sequencing information to messages themselves).
SignalR offers a limited number of client SDKs: C#, Java, Python, and JavaScript. There are no SDKs for platforms and languages like iOS, Android, Go, Ruby or PHP.
SignalR is designed to be a single-region solution, which can negatively impact its performance, reliability, and availability.
Scaling SignalR (or any other WebSocket-based implementation) yourself is likely to be difficult, expensive, and time-consuming. Read about the challenges of scaling SignalR. You can abstract away the pain of managing SignalR yourself by using the managed version, Azure SignalR Service. However, SignalR Service also has its limitations; for example, it only provides a maximum 99.95% uptime guarantee (for premium accounts), which amounts to almost 4.5 hours of allowed downtime / unavailability per year. This SLA might not be reliable enough for critical use cases, like healthcare apps that must be available 24/7. The situation is worse for non-premium accounts, where the SLA provided is 99.9% (almost 9 hours).
SignalR vs WebSocket: what are the key differences?
WebSocket is an event-driven protocol that enables bidirectional, full-duplex communication between client and server. In contrast, SignalR is a solution that provides an abstraction layer on top of WebSockets, making it easier and faster to add realtime web functionality to apps.
SignalR provides features such as automatic reconnections, fallback transports, or the ability to broadcast messages to all connected clients, or only to specific groups of clients. With vanilla WebSockets, you don’t benefit from such features out of the box - you have to build all these capabilities yourself if they're relevant to your use case.
Another significant difference: the WebSocket protocol is a widely adopted standard - it’s supported across most programming languages, and almost all modern browsers natively support the WebSockets API. In comparison, SignalR offers a limited number of SDKs, for languages like C# (.NET), JavaScript, or Java. There are no official SDKs for platforms like iOS, Go, Ruby, or PHP.
Finally, a word on performance. As SignalR is a more complex (and demanding) solution than raw WebSockets, it’s safe to expect some performance-related differences. For example, it takes less time to establish a raw WebSocket connection than a SignalR connection. SignalR almost certainly has higher memory and CPU requirements than vanilla WebSockets.
Should you use SignalR or WebSocket?
Whether you should use SignalR or WebSocket depends on a couple of factors. Firstly, the specifics of your use case. For example, raw WebSocket is suitable for PoCs and simplistic scenarios, such as pushing data to browsers. On the other hand, for more complex use cases, such as a production-ready chat app, SignalR is a superior alternative, as it allows you to move faster than plain WebSockets, and provides features like automatic reconnections and the ability to broadcast messages to multiple clients (useful for multi-user chat).
Another thing to bear in mind is the degree of flexibility you need for your use case. Raw WebSockets provide more flexibility - you can design your own architecture and your own protocol on top, using essentially any programming language you want - although this is incredibly difficult and time-consuming. In comparison, SignalR is available as a fully managed service (in addition to the open-source version), which removes the need to provision and manage infrastructure in-house. However, SignalR has a pre-designed architecture and limited platform support, which may become restrictive at some point.
SignalR and Websocket alternatives
While they both have their advantages, neither SignalR nor WebSockets provide a complete realtime messaging solution.
With SignalR, you have to use ASP.NET on the server, and there’s still a lot of work and ongoing maintenance to ensure your SignalR instance scales smoothly with your application.
As you enter a production environment, you might start to notice gaps in SignalR’s quality of service (even when using the hosted Azure version). For example, SignalR does not guarantee message ordering or exactly-once delivery.
Since WebSockets are a lower-level protocol, they can’t boast to solve any of the above problems. But they are more flexible, in case you want to give your messaging layer a very specific shape or optimize performance.
We’ve written about some alternative open source WebSocket libraries as well as SignalR alternatives to investigate, but if you want to avoid the headache and high costs of managing WEbSocket infrastructure in house, the solution is clear.
Enter Ably.
Ably: A fully-featured and scalable alternative to SignalR and WebSockets
If you’re looking for a more robust alternative to SignalR, we invite you to explore the Ably platform. See how SignalR compares to Ably or how WebSockets compare to Ably.
Ably provides globally-distributed edge infrastructure and hosted WebSocket APIs to build and deliver realtime features like multiplayer games, chat, and location tracking at internet scale.
Our platform offers robust capabilities and guarantees, including 25+ client SDKs targeting every major platform and programming language, feature-rich Pub/Sub messaging, guaranteed message ordering and delivery, and a 99.999% uptime SLA.
To find out more about what Ably can do for you, get started with a free account.
SignalR vs WebSocket FAQ
What is the difference between SignalR and WebSocket?
WebSocket is a communication protocol that enables bidirectional, message-oriented streaming of text and binary data between client and server. Meanwhile, SignalR is a library built on top of WebSockets that simplifies the process of adding realtime functionality to websites.
Why would I use SignalR over calling WebSockets directly?
SignalR provides features you’d otherwise have to implement yourself with WebSockets. Additionally, SignalR has a fallback to HTTP polling in case the WebSocket connection can’t be established. It’s usually quicker to get started with, even though it’s less flexible in the long-term.
Is SignalR better than WebSocket?
Depending on your requirements, SignalR might be quicker to get started with than WebSockets due to the fact it’s a library rather than a protocol, but that doesn’t necessarily mean it’s superior. SignalR might not be as performant as WebSockets in some cases, and the reliance on ASP.NET for self-hosting means it’s unsuitable backend developers not using the .NET stack.
Is SignalR still supported?
ASP.NET Core SignalR and Azure’s SignalR service are still actively supported. The original ASP.NET SignalR library is in maintenance mode, meaning new features aren’t considered, but bug fixes may be released.
Recommended Articles
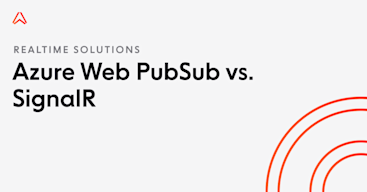
Azure Web PubSub vs SignalR: Which one is best for you?
SignalR and Azure Web PubSub are often used to build realtime apps like chat. Discover their differences and similarities, and compare their features.

Pusher vs. SignalR: feature comparison, use cases, pros and cons
Learn how Pusher and SignalR are different, compare their features, understand their pros and cons, and discover alternative solutions you can use to build realtime functionality.