- Topics
- /
- Realtime technologies
- /
- SignalR deep dive: Key concepts, use cases, and limitations
SignalR Deep Dive: How It Works, Use Cases, and Limitations
SignalR is an open-source library for ASP.NET that enables realtime communication between servers and clients. It abstracts away the complexities of connection management and messaging, letting you push updates instantly without polling or manual socket handling.
In this guide, we’ll explore how SignalR works, examine real-world use cases, cover common best practices, and identify its limitations — particularly when it comes to scalability and performance in production systems.
How does SignalR work?
SignalR is an event-driven technology that enables realtime features in .NET applications by managing persistent connections between the server and connected clients. It negotiates the most efficient transport available, prioritizing WebSockets, and falling back to Server-Sent Events (SSE) or long polling when necessary.
Under the hood, SignalR handles:
Transport negotiation: Automatically selects the best supported transport between client and server — prioritizing WebSockets, and falling back to SSE or long polling as needed.
Hubs: Provides an abstraction for client-server communication using remote procedure calls (RPC), allowing server methods to be invoked directly from client-side code and vice versa.
Connection state: Tracks individual client connections and supports grouping users (e.g. by role, room, or session) for targeted message delivery (must be manually managed).
Fallback logic: Gracefully degrades when the preferred transport is unavailable, ensuring that clients can still connect in constrained environments.
Cross-platform client support: Offers client libraries for .NET, JavaScript, Java, and other platforms, making it suitable for web, desktop, and mobile real-time apps across varied environments.
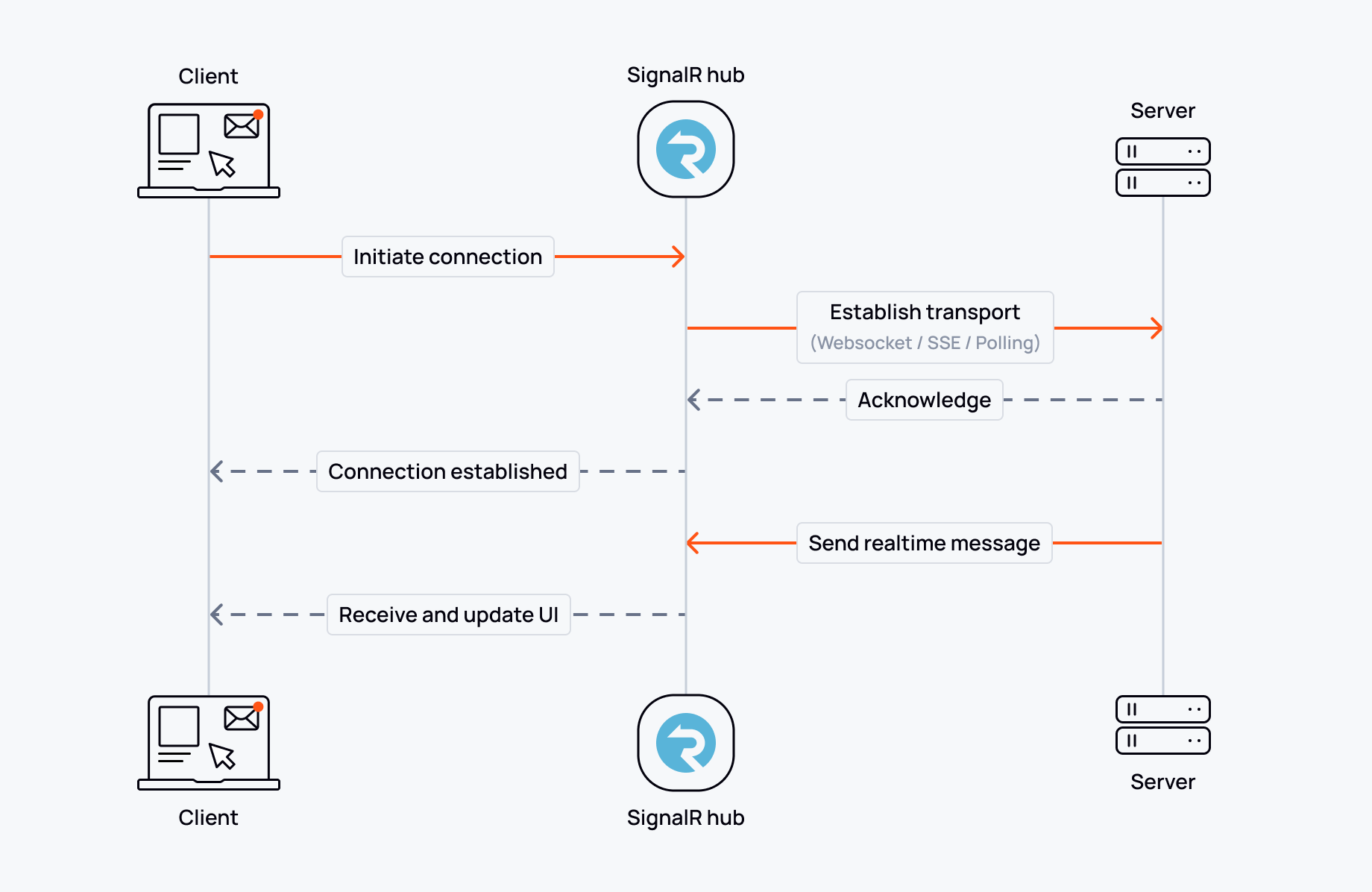
SignalR use cases
SignalR is ideal for realtime communication features in apps built within the .NET ecosystem. Common use cases include:
Live dashboards for analytics or trading
In-app chat for customer service or gaming
Collaborative tools like document editors or whiteboards
Instant notifications in web or mobile UIs
Multiplayer games requiring low-latency interactions
While SignalR is a powerful tool for quick-to-market features, it comes with architectural tradeoffs when scaling to global or high-throughput environments.
SignalR best practices
To make the most of SignalR in production deployments, consider the following best practices:
Use WebSockets for performance: WebSockets offer the most efficient, low-latency connection. SignalR uses them by default when available. Ensure your infrastructure — including proxies, firewalls, and client environments — supports WebSocket upgrades.
Enable automatic reconnection: In ASP.NET Core SignalR, reconnection is not enabled by default. Use
.withAutomaticReconnect()
in your client code to restore connectivity after dropouts. Reconnection does not automatically restore group membership — this must be handled manually.Use a backplane to scale out: SignalR does not share connection state between servers out of the box. Use a Redis backplane or Azure SignalR Service to coordinate messaging across multiple app instances.
Manually manage group membership: SignalR supports groups, but group management is manual. Use
AddToGroupAsync()
andRemoveFromGroupAsync()
in your Hub logic. Reassign users to groups on reconnection.Keep messages lightweight: Avoid large payloads and high-frequency messages. Use compact formats like JSON, and throttle or debounce high-frequency events (e.g. typing, mouse movement) to reduce bandwidth and load.
Add observability and logging: SignalR provides limited native telemetry. Log connection attempts, disconnections, transport types, and message failures. Integrate with observability platforms like Application Insights, Datadog, or Prometheus.
Handle disconnects gracefully: Network interruptions are common. Display UI indicators for disconnected or reconnecting users. Queue or delay actions until the connection is restored. Clean up server-side resources using
OnDisconnectedAsync()
.Secure your SignalR endpoints: Use JWTs or claims-based authentication. Protect hubs and methods using
[Authorize]
. SignalR authenticates only once at connection time - you should still validate every incoming message.Version your hub APIs: Hub methods are tightly coupled to client code. Use versioned hub names (e.g.
ChatHubV2
) to avoid breaking changes for existing clients when evolving your API.Test under load and poor network conditions: Simulate network dropouts, high user concurrency, and transport fallback behavior. Use tools like k6 or Artillery to verify performance and resilience under real-world stress.
SignalR limitations
SignalR is well-suited for simple realtime needs in .NET applications, but it presents several architectural constraints when scaling beyond a small number of concurrent users or distributed environments.
1. Server-bound connection state
⚠️ Problem: SignalR connections are stateful and tied to individual servers.
Impact: Messages can't reach clients connected to other servers in a scaled-out deployment.
🛠️ What this means: You’ll need to implement a Redis backplane or use Azure SignalR Service to share state and messages across instances.
2. No native message durability or delivery guarantees
⚠️ Problem: SignalR doesn’t persist or retry messages.
📉 Impact: Clients that disconnect during transmission lose messages permanently.
🛠️ What this means: To ensure reliability, you’ll need to layer in your own queueing and retry mechanism.
3. Backplane bottlenecks under load
⚠️ Problem: Redis or SQL backplanes become bottlenecks under high throughput or fan-out scenarios.
📉 Impact: Performance degrades as message volume or connection count grows.
🛠️ What this means: You’ll need to carefully monitor Redis performance and consider horizontal scaling, which increases ops complexity.
4. Limited observability and diagnostics
⚠️ Problem: SignalR lacks built-in monitoring for transport fallback, dropped messages, or latency.
📉 Impact: Diagnosing issues in production is difficult and reactive.
🛠️ What this means: You'll need to build your own telemetry and integrate third-party monitoring tools.
5. No global edge or multi-region infrastructure
⚠️ Problem: All SignalR traffic flows through a single region or server cluster.
📉 Impact: Users located far from the server will experience higher latency.
🛠️ What this means: You’ll need to manually design a geo-distributed architecture if low-latency global performance is critical.
6. Transport fallback behavior can degrade UX
⚠️ Problem: SignalR silently falls back to long polling or SSE if WebSockets aren’t available.
📉 Impact: Performance may suffer without visibility into the fallback behavior.
🛠️ What this means: You should log and monitor transport types used and proactively design for fallback scenarios.
7. High per-connection resource cost
⚠️ Problem: Each SignalR connection consumes server memory, threads, and CPU.
📉 Impact: Supporting high concurrency becomes difficult without tuning.
🛠️ What this means: Optimize server configuration and consider moving to managed solutions if your connection count grows.
8. Limited SDK support
⚠️ Problem: SignalR only supports .NET, JavaScript, and Java officially.
📉 Impact: Other platforms like Python, Go, Swift, Flutter, or PHP require custom solutions.
🛠️ What this means: You'll need to write and maintain your own WebSocket clients or translation layers.
9. Limited SLA for Azure SignalR Service
⚠️ Problem: Azure SignalR offers a 99.95% SLA for premium plans (4.5 hours/year of downtime), and 99.9% for others (9 hours/year).
📉 Impact: These uptime guarantees may not be sufficient for healthcare, finance, or other mission-critical sectors.
🛠️ What this means: You need to assess SLA fit and plan fallback or redundancy strategies accordingly.
10. Self-hosting SignalR is complex and costly
⚠️ Problem: Running your own SignalR stack involves managing scaling, Redis, failover, and observability.
📉 Impact: Significant time and budget must be invested to match the reliability of managed platforms.
🛠️ What this means: Expect high engineering and infrastructure costs. Benchmark data shows that 65% of self-hosted WebSocket systems have outages each 12-18 months, it takes 10.2 person-months to build a minimal realtime system in-house, and $100-$200k per year in upkeep is common.
Summary: Key scalability constraints
Limitation | Scalability impact |
---|---|
Server-bound connection state | Limits horizontal scaling |
Requires backplane | Adds latency and complexity |
No message durability | Messages can be lost |
Minimal observability | Difficult to monitor or debug |
No edge or global routing | Poor experience for remote users |
High per-connection resource cost | Limits concurrency |
.NET-only architecture | Harder to scale in polygot systems |
Modern alternatives to SignalR
While SignalR is well-suited for many real-time use cases within the Microsoft ecosystem, especially for applications already built on ASP.NET Core, there are scenarios where alternative approaches may be a better fit — particularly when scale, cross-platform support, or global delivery are critical.
Here are some common reasons teams consider alternative realtime platforms:
Global reach: If your users are distributed across multiple regions, you may need a platform with built-in edge acceleration or multi-region infrastructure to reduce latency.
Horizontal scalability: Scaling SignalR requires Redis backplanes or managed services. Alternatives may offer native clustering or pub/sub architectures that scale more easily.
Polyglot environments: Teams building across multiple languages and platforms may prefer solutions with first-class SDKs for web, mobile, and backend services.
Operational simplicity: Fully managed pub/sub platforms handle infrastructure concerns like connection management, failover, observability, and message guarantees out of the box.
Advanced delivery features: Some platforms offer built-in support for message persistence, history, guaranteed delivery, and fine-grained access controls.
Examples of alternative platforms
Ably – A fully managed, globally distributed pub/sub platform with support for WebSockets, message ordering, delivery guarantees, and 25+ client SDKs. Built for scale, with enterprise-grade SLAs and observability. See how Ably compares to SignalR.
Azure Web PubSub – A cloud-native service from Microsoft that offers real-time messaging similar to SignalR, but with enhanced scalability and tighter integration with Azure services.
MQTT brokers (e.g., HiveMQ, EMQX) – Best suited for IoT and telemetry use cases that require efficient binary protocols and topic-based routing. MQTT platforms offer features like persistent sessions, QoS levels, and retained messages.
WebSocket infrastructure providers – For teams that need full control over the stack, platforms like Cloudflare, AWS API Gateway (WebSockets), or custom NGINX/HAProxy setups can serve as a foundation. These options typically require more engineering investment and maintenance.
The best choice depends on your system architecture, development stack, and operational requirements. SignalR remains a strong option for many .NET-centric applications, but other platforms may offer greater flexibility, scale, and resilience for cross-platform or mission-critical use cases.
Recommended Articles
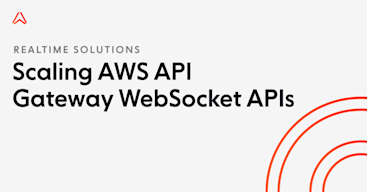
Scaling AWS API Gateway WebSocket APIs - what you need to consider
AWS API Gateway WebSocket APIs allow you to power realtime communication for use cases like chat and live dashboards. But how well does AWS API Gateway scale?
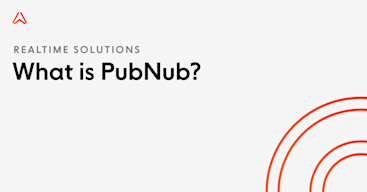
Guide to PubNub: What it is, how it works and when to use it
PubNub is a platform for building realtime applications. Discover what it does, how it works, its use cases, limitations, and suitable alternatives.
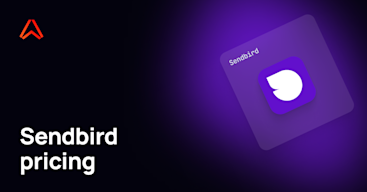
Sendbird pricing: What you need to know
Explore how Sendbird pricing works, including their MAU pricing model, tiers, and how to decide if it is right for you.