Ably Pub/Sub
When realtime matters, developers choose Ably Pub/Sub
Flexible APIs for building any live and interactive experience. Powered by Ably's scalable and reliable WebSocket infrastructure.
Quick start docs
Build
Develop rapidly using Ably Pub/Sub’s simple APIs. Combine with our other products to deliver the richest user experience.
Integrate
Increase developer productivity and shorten delivery timelines. Fast track integration with your current and future tech stack.
Scale
Brands trust Ably Pub/Sub to power their critical applications - autoscaling to billions of devices with trillions of operations each month.
Deliver
Built to serve the world’s most demanding realtime workloads, providing 5+ years of 100% uptime.
Building blocks for realtime experiences
Everything. Everywhere. All in realtime.
Level-up your realtime application with Ably Pub/Sub’s extensive feature set.
Channels
Organize messages into different topics and enable clients to subscribe and publish messages to them.
View docsRewind
Choose a point in the past at which a client joins a channel, so that they can receive messages published before they joined.
View docsMessage persistence
Persist messages published on channels and retrieve them via our history APIs.
View docsConnection state and recovery
Automatically re-establish failed connections and replay missed messages.
View docsMessage ordering
Guaranteed message ordering from any single realtime or non-realtime publisher to all subscribers.
View docsExactly once delivery
Publish idempotency enables us to offer exactly-once message delivery guarantees.
View docsMessage batching
Coming soon! Automatically optimize costs and enhance performance in large-scale, high-throughput applications.
View docsBandwidth efficient deltas
Significant bandwidth reduction when broadcasting changes using binary Delta compression.
View docsSubscription filters
Selectively subscribe to messages on a channel using filter expressions.
View docsPush notifications
Deliver cross-platform and web push notifications with our iOS and Android SDKs.
View docsSystem metadata
Find out which channels are popular, where users are, or receive realtime notifications as changes happen.
View docsDynamic channel groups
Automatically subscribe to multiple active channels simultaneously that match your specified criteria.
Consumer groups
Create sets of consumers that cooperate to consume data from certain channels.
Channels
Organize messages into different topics and enable clients to subscribe and publish messages to them.
View docsRewind
Choose a point in the past at which a client joins a channel, so that they can receive messages published before they joined.
View docsMessage persistence
Persist messages published on channels and retrieve them via our history APIs.
View docsConnection state and recovery
Automatically re-establish failed connections and replay missed messages.
View docsMessage ordering
Guaranteed message ordering from any single realtime or non-realtime publisher to all subscribers.
View docsExactly once delivery
Publish idempotency enables us to offer exactly-once message delivery guarantees.
View docsMessage batching
Coming soon! Automatically optimize costs and enhance performance in large-scale, high-throughput applications.
View docsBandwidth efficient deltas
Significant bandwidth reduction when broadcasting changes using binary Delta compression.
View docsSubscription filters
Selectively subscribe to messages on a channel using filter expressions.
View docsPush notifications
Deliver cross-platform and web push notifications with our iOS and Android SDKs.
View docsSystem metadata
Find out which channels are popular, where users are, or receive realtime notifications as changes happen.
View docsDynamic channel groups
Automatically subscribe to multiple active channels simultaneously that match your specified criteria.
Consumer groups
Create sets of consumers that cooperate to consume data from certain channels.
And so much more...
- Multiple protocol support - Websockets, MQTT, HTPP and SSE
- Bandwidth efficient binary protocol support
- Channel connection rate limiting and slow mode
- Token revocation and user management
- Message queues for scalable server-side message consumption
- End-to-end encryption
choose your platform
Crafted SDKs for popular platforms
Ably's SDKs provide a consistent and idiomatic API across a variety of supported platforms.
JavaScript
React
Python
Java
.NET
Ruby
JavaScript
React
Python
Java
.NET
Ruby
JavaScript
React
Python
Java
.NET
Ruby
Flutter
Obj-C
Swift
PHP
Go
C#.NET
Flutter
Obj-C
Swift
PHP
Go
C#.NET
Flutter
Obj-C
Swift
PHP
Go
C#.NET
integrate with other services
Easy integration, whatever your stack
Connect with pre-built integrations for Webhooks, Lambdas, databases, cloud services, observability tools and stream processors.
Amazon Lambda functions
Azure functions
Cloudflare Workers
Google Cloud functions
AMQP
Amazon Kinesis
Amazon Lambda functions
Azure functions
Cloudflare Workers
Google Cloud functions
AMQP
Amazon Kinesis
Amazon Lambda functions
Azure functions
Cloudflare Workers
Google Cloud functions
AMQP
Amazon Kinesis
Amazon SQS
Kafka
IFTTT
Zapier
Apache Pulsar
Terraform
Amazon SQS
Kafka
IFTTT
Zapier
Apache Pulsar
Terraform
Amazon SQS
Kafka
IFTTT
Zapier
Apache Pulsar
Terraform
Composable realtime
Combine Ably products to build the realtime experiences you want.
Select a product below to see some example combinations:
Ably
PubSub
Low-level APIs to build any realtime experience
Ably
Chat
Rapidly build chat features and roll-out at scale
Ably
Spaces
Create collaborative environments in a few lines of code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
/* Create an Ably Pub/Sub client */
let realtimeClient = new Ably.Realtime(clientOptions)
/* Subscribe to and publish comments */
let commentsChannel = realtimeClient.channels.get('comments')
commentsChannel.subscribe((msg) => alert(`New comment: ${msg.data}`))
commentBtn.on('click', () => commentsChannel.publish(commentField.value))
/* Compose a richer collaborative realtime experience with Ably Spaces */
let spacesClient = new Spaces(realtimeClient)
let space = spacesClient.get(currentDocumentId)
/* Show cursor position */
space.cursors.subscribe('update', (cursorUpdate) => {
console.log(`${cursorUpdate.clientId} moved to ${cursorUpdate.position}`)
})
/* Share mouse movement updates with other collaborators */
window.addEventListener('mousemove', ({ X, Y }) => {
space.cursors.set({ position: { x: X, y: Y } })
})
Ably
LiveSync
Sync database changes with frontend clients
Ably
Asset Tracking
Simple APIs to build realtime tracking applications
Ably
LiveObjects
Sync application state across multiple devices
get inspired
Ably Pub/Sub in action
Discover how top brands leverage Ably Pub/Sub to build realtime experiences for a global user base.
Customer support
HubSpot enables 128,000 businesses with live chat that just works.
Read customer story
Fan engagement
NASCAR relies on Ably to deliver live experiences for millions of race fans worldwide.
Read customer story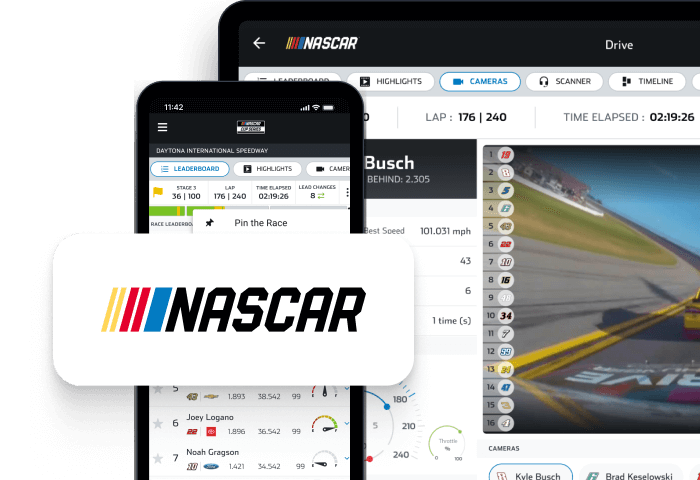
Data broadcast
Genius Sports slashes cost and lowers latencies for last-mile live sports data delivery.
Read customer story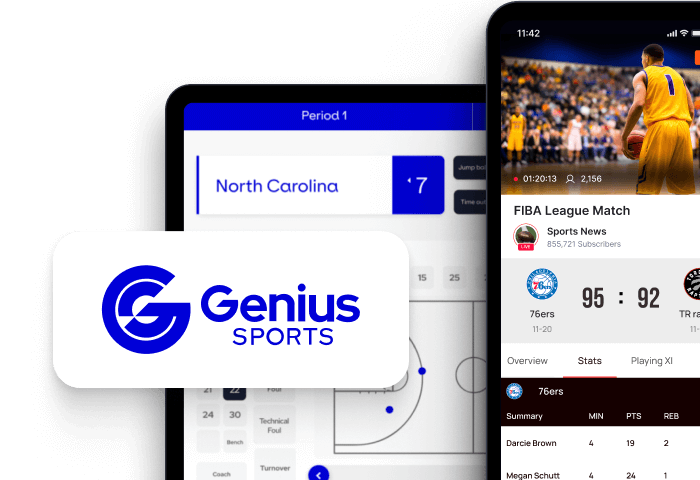
Pricing built for scale
Ably not only enables you to make the most of your budget, but also enjoy greater flexibility, faster build times and a quicker ROI.
Usage-based pricing
With billing by-the-minute, our pricing gives you granular control over your costs.
Volume discounts
The more you use the platform, the lower your overall cost becomes.
Full transparency
We bill for connections, messages and channels. Nothing more.
Cost optimization
We maximize your ROI by actively designing platform features targeted at reducing consumption.
When every message counts
Designed for enterprise
Get unmatched expertise paired with a host of value-adding options to keep your business-critical applications running at optimal performance.
HIPAA BAA
Compliance for your healthcare solution.
Premium support
24x7 tech support and incident management with sub 15 mins response times.
Firehose integrations
Available for Kafka, Pulsar and Kinesis.
Architectural guidance
Expert advice on how to balance cost and user-experience for your use-cases.
Dedicated cluster
Dedicated capacity in cloud infrastructure including networking resources and servers.
99.999% uptime
Uptime guarantee with SLA.
US/EU routing of data
Choose where your data is stored.
CNAME
Custom CNAME endpoints.
SSO and SCIM provisioning
Choose your own authentication provider.
SOCII
Information security compliance
Limitless capacity
Scale to billions.
Enterprise observability and analytics
Get the data and insights you need, including access to our Datadog integration.
engineered to perform
The definitive realtime experience platform of the internet
Performance
We focus on predictability of latencies to provide certainty in uncertain operating conditions.
<50ms
global median latency
19M+
concurrent connections
Reliability
Designed to preserve continuity of service we ensure sufficient redundancy at a regional and global level.
5+ years
since last outage
99.999999%
message survivability
Scale
Elastic and highly-available. Ably is built to handle extreme scale and high concurrent connections.
1B+
connected devices per month
2T+
API operations per month
Network
Truly distributed global edge network. Delivers a globally consistent experience to users.
635
points of presence
11
globally distributed regions