In light of the recent deprecation of MongoDB Atlas Device Sync (ADS), developers are seeking alternative solutions to synchronize on-device data with cloud databases. Ably LiveSync offers a potential alternative and can replace some of ADS’s functionality, enabling realtime synchronization of database changes to devices at scale. LiveSync allows for a large number of changes to MongoDB to be propagated to end user devices in realtime and store the changes in any number of local storage options - from an embedded database to in-memory storage.
For instance, imagine an inventory app that needs to broadcast stock updates to multiple devices in realtime. Ably LiveSync allows you to automatically subscribe to inventory changes in your database and broadcast this data to millions of clients at scale, allowing them to remain synchronized with the state of your inventory in realtime.
This article explains why on-device storage is critical, explores existing solutions, and demonstrates how Ably LiveSync’s MongoDB connector can help with a brief code tutorial.
Why keep information on-device?
Local storage is a must for apps that need offline access or fast performance— like e-commerce inventory apps, or news apps downloading content for offline browsing. But not every app needs it. If your app is always online or just streams read-only data, you can skip the complexity of a local database. Thankfully, with Ably, you can adapt to your use case, whether you need offline support or just realtime updates. Some of the benefits of on-device storage are:
- Offline access: Storing data directly on the device ensures users can seamlessly access and interact with information even when they have no internet connection or are in areas with poor connectivity. This is particularly crucial for users who frequently work in offline environments or travel to locations with unreliable network coverage.
- Performance: Applications demonstrate significantly improved response times and reduced latency when accessing data stored locally, as opposed to making time-consuming server calls across the network. This local data access eliminates network-related delays and provides instantaneous data retrieval for critical operations.
- Cost efficiency: Users experience substantial savings on their data usage and associated costs since the application doesn't need to repeatedly download information from remote servers. This is especially beneficial for users with limited data plans or in regions where mobile data is expensive.
- User experience: Users benefit from a consistently smooth and reliable application experience, maintaining uninterrupted access to their data regardless of their network status or connection quality. This reliability helps build user trust and satisfaction with the application.
Options for storing information on device
Modern mobile operating systems provide a variety of ways to store information on device:
- iOS: Includes UserDefaults, CoreData, and SQLite, with flexibility for additional solutions based on specific needs.
- Android: Provides shared preferences, Room database, and file storage.
- Cross-platform frameworks: With React Native, react-native-async-storage is a popular starting library for simple needs. However, for advanced use cases requiring NoSQL-like abilities, some good choices here would be RealmDB (which, unfortunately, as we know, is being deprecated), UnQLite, LevelDB, or Couchbase.
Regardless of your choice of an on-device database and storage methodology, you can use Ably LiveSync to synchronize data from your managed or on-premises database to mobile devices in realtime. This includes MongoDB - as well as Atlas. While we currently support only MongoDB and PostgresSQL, we are working on adding support for other database engines.
What is Ably LiveSync?
Ably LiveSync lets you monitor database changes and reliably broadcast them to millions of frontend clients, keeping them up-to-date in realtime.
LiveSync works with any tech stack and prevents data inconsistencies from dual-writes while avoiding scaling issues from "thundering herds" — sudden surges of traffic that can overwhelm your database.
How to persist data locally with Ably
Let’s explore how to build a simple in-store management app that tracks product inventory using React Native and SQLite for local storage and a Mongo Atlas for our cloud database. Despite Mongo being a document storage and SQLite being a relational database, the two can be used in combination. We are going to use the Ably SDK callback methods to store documents and changes inside our local SQLite database.
Setting up Ably
For simplicity, we’ll stick to TypeScript. Before anything else, create a new React Native project using the CLI:
npx @react-native-community/cli@latest init AwesomeStore
Creating a MongoDB integration rule with Ably
Now we need to create a new channel that streams database changes to your clients. This ensures realtime updates whenever your MongoDB data changes. To create an integration rule that will sync your MongoDB database with Ably, you’ll first have to sign up for an Ably account.
Once that’s done, you should have access to your Ably dashboard. Create an app or select the app you wish to use. Navigate to the Integrations tab > Create a new integration rule > MongoDB. Fill out the Connection URL with your MongoDB connection URL; Database name with your db name (for this example, SQLiteDatabase
); and Collection with your collection name (for this example, products
). For more information on this process and the parameters involved, check out our docs.
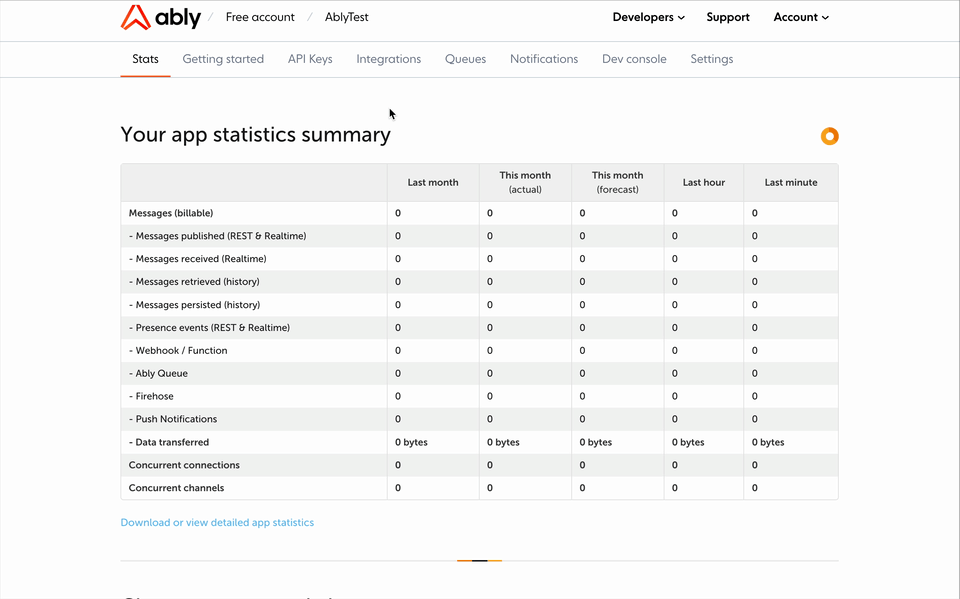
This sets up a new channel, built on top of our core Ably Pub/Sub product, which streams changes (through MongoDB change streams) from your database to your clients.This essentially ensures that any change that occur in your database will be delivered to any device subscribed to a channel.
Creating the local datastore
We’ll create a new file in our project called datastore.js
and initialize SQLite:
export const createTables = async (db: SQLiteDatabase) => {
const query = `CREATE TABLE IF NOT EXISTS products(
id INT32
name TEXT NOT NULL
description TEXT
quantity INT32
);`;
await db.executeSql(query);
};
After the tables are created, we need a way to retrieve store products and update their stock:
export const getProducts = async (db: SQLiteDatabase): Promise<ToDoItem[]> => {
try {
const products: StoreProduct[] = [];
const results = await db.executeSql(`SELECT id, name, description, quantity FROM ${tableName}`);
results.forEach(result => {
for (let index = 0; index < result.rows.length; index++) {
products.push(result.rows.item(index))
}
});
return products;
} catch (error) {
console.error(error);
throw Error('Failed to get products!');
}
};
export const saveOrUpdateProducts = async (db: SQLiteDatabase, products: StoreProduct[]) => {
const insertQuery =
`INSERT OR REPLACE INTO ${tableName}(id, name, description, quantity) values` +
products.map(i => `(${i.id}, '${i.value}', '${i.description}', '${i.quantity}')`).join(',');
return db.executeSql(insertQuery);
};
Receiving database changes from MongoDB over Ably
Let’s take a look at how we can receive changes from the configured Ably channel. More information can be found in our documentation, but this is the important snippet we need - setting up the Ably Realtime SDK:
import * as Ably from 'ably';
// Instantiate the Realtime SDK
const ably = new Ably.Realtime('[your API key]');
// Get the channel to subscribe to
const channel = ably.channels.get('store:1');
// Subscribe to messages on the 'store:1' channel
await channel.subscribe((message) => {
// Print every change detected in the channel
console.log('Received a change event in realtime: ' + message.data)
});
We need to write a new function that will take the payload of message.data
and store it in our database:
const addOrUpdateProduct = async (data: any) => {
try {
let product: StoreProduct = {
id: data.fullDocument.id,
name: data.fullDocument.name,
description: data.fullDocument.description,
quantity: data.fullDocument.quantity,
}
const db = await getDBConnection();
await saveOrUpdateProducts(db, product);
} catch (error) {
console.error(error);
}
};
We can call our new function in our message subscription:
// Subscribe to messages on the 'store:1' channel
await channel.subscribe((message) => {
// Print every change detected in the channel
console.log('Received a change event in realtime: ' + message.data)
if (data.ns.coll === "products") {
await addOrUpdateProduct(message.data)
} else if (data.ns.coll === "store") {
// Another function which updates changes for the `store` collection
} else {
console.warn("Unknown collection");
}
});
The full workflow
With this setup, the app listens for realtime updates from your MongoDB collection and persists changes locally, ensuring an up-to-date inventory system even when offline.
Final thoughts: What makes Ably different
I hope that gives you a good overview of what Ably LiveSync’s MongoDB Connector can do! Besides providing a potential alternative to Atlas Device Sync, Ably, as a realtime communications platform, is built for scalability and reliability. Here are some features of Ably Pub/Sub, the backbone upon which LiveSync’s database connector is built:
- Predictable performance: A low-latency and high-throughput global edge network, with median latencies of <50ms.
- Guaranteed ordering & delivery: Messages are delivered in order and exactly once, even after disconnections.
- Fault-tolerant infrastructure: Redundancy at regional and global levels with 99.999% uptime SLAs. 99.999999% (8x9s) message availability and survivability, even with datacenter failures.
- High scalability & availability: Built and battle-tested to handle millions of concurrent connections at scale.
- Optimized build times and costs: Deployments typically see a 21x lower cost and upwards of $1M saved in the first year.
Try Ably today and explore our MongoDB connector.